line 86, in test_data x_test = np.reshape(x_test, (x_test.shape[0], x_test.shape[1], 5)) IndexError: tuple index out of range应该如何修改
时间: 2024-01-13 18:05:32 浏览: 154
这个错误通常是由于 x_test 的形状不是你期望的形状所导致的。你需要检查一下 x_test 的形状,看看它的实际形状是否与你所期望的形状相同。你可以使用 print(x_test.shape) 命令来检查 x_test 的形状。
如果你发现 x_test 的形状不正确,你可以使用 np.expand_dims(x_test, axis) 命令来为 x_test 添加一个新维度。其中,axis 是你想要添加的新维度的位置。例如,如果你想要将 x_test 转换为形状为 (n_samples, n_timesteps, 5) 的张量,你可以使用以下代码:
```
x_test = np.expand_dims(x_test, axis=1)
x_test = np.reshape(x_test, (x_test.shape[0], x_test.shape[2], 5))
```
这将在第二个维度上添加一个新维度,并将 x_test 转换为所需的形状。
相关问题
line 86, in test_data x_test = np.reshape(x_test, (x_test.shape[0], x_test.shape[1], 5)) IndexError: tuple index out of range
这个错误的意思是你试图访问一个元组中不存在的索引。在这种情况下,你正在尝试使用 x_test.shape[1] 访问一个形状为 (x_test.shape[0],) 的元组的第二个元素。这意味着 x_test 只有一个维度,而你试图将它重塑为一个形状为 (x_test.shape[0], x_test.shape[1], 5) 的三维数组,其中第二个维度的大小为 x_test.shape[1]。你需要检查一下你的代码,看看为什么 x_test 的形状不是你期望的。你可以使用 print(x_test.shape) 来打印 x_test 的形状,以便找出问题所在。
import numpy as np import pandas as pd import matplotlib.pyplot as plt from sklearn.preprocessing import MinMaxScaler from keras.models import Sequential from keras.layers import Dense, LSTM from sklearn.metrics import r2_score,median_absolute_error,mean_absolute_error # 读取数据 data = pd.read_csv(r'C:/Users/Ljimmy/Desktop/yyqc/peijian/销量数据rnn.csv') # 取出特征参数 X = data.iloc[:,2:].values # 数据归一化 scaler = MinMaxScaler(feature_range=(0, 1)) X[:, 0] = scaler.fit_transform(X[:, 0].reshape(-1, 1)).flatten() #X = scaler.fit_transform(X) #scaler.fit(X) #X = scaler.transform(X) # 划分训练集和测试集 train_size = int(len(X) * 0.8) test_size = len(X) - train_size train, test = X[0:train_size, :], X[train_size:len(X), :] # 转换为监督学习问题 def create_dataset(dataset, look_back=1): X, Y = [], [] for i in range(len(dataset) - look_back - 1): a = dataset[i:(i + look_back), :] X.append(a) Y.append(dataset[i + look_back, 0]) return np.array(X), np.array(Y) look_back = 12 X_train, Y_train = create_dataset(train, look_back) #Y_train = train[:, 2:] # 取第三列及以后的数据 X_test, Y_test = create_dataset(test, look_back) #Y_test = test[:, 2:] # 取第三列及以后的数据 # 转换为3D张量 X_train = np.reshape(X_train, (X_train.shape[0], X_train.shape[1], 1)) X_test = np.reshape(X_test, (X_test.shape[0], X_test.shape[1], 1)) # 构建LSTM模型 model = Sequential() model.add(LSTM(units=50, return_sequences=True, input_shape=(X_train.shape[1], 1))) model.add(LSTM(units=50)) model.add(Dense(units=1)) model.compile(loss='mean_squared_error', optimizer='adam') model.fit(X_train, Y_train, epochs=5, batch_size=32) #model.fit(X_train, Y_train.reshape(Y_train.shape[0], 1), epochs=10, batch_size=32) # 预测下一个月的销量 last_month_sales = data.tail(12).iloc[:,2:].values #last_month_sales = data.tail(1)[:,2:].values last_month_sales = scaler.transform(last_month_sales) last_month_sales = np.reshape(last_month_sales, (1, look_back, 1)) next_month_sales = model.predict(last_month_sales) next_month_sales = scaler.inverse_transform(next_month_sales) print('Next month sales: %.0f' % next_month_sales[0][0]) # 计算RMSE误差 rmse = np.sqrt(np.mean((next_month_sales - last_month_sales) ** 2)) print('Test RMSE: %.3f' % rmse)IndexError Traceback (most recent call last) Cell In[1], line 36 33 X_test, Y_test = create_dataset(test, look_back) 34 #Y_test = test[:, 2:] # 取第三列及以后的数据 35 # 转换为3D张量 ---> 36 X_train = np.reshape(X_train, (X_train.shape[0], X_train.shape[1], 1)) 37 X_test = np.reshape(X_test, (X_test.shape[0], X_test.shape[1], 1)) 38 # 构建LSTM模型 IndexError: tuple index out of range
这个错误提示是因为在第36行中,X_train数组的维度不符合要求。具体地说,X_train的维度为 (样本数量, 时间步长, 特征数),而在代码中reshape时将其调整为了 (样本数量, 时间步长, 1),这表示只有一个特征,但实际上在读取数据时有多个特征。
可能的解决方法是检查数据读取部分是否正确,确保X数组包含了所有的特征,并且在构建数据集和LSTM模型时正确地设置了特征数和时间步长等参数。
阅读全文
相关推荐
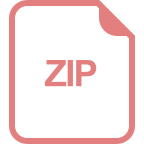
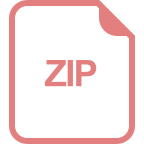
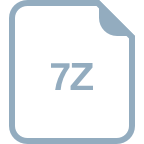












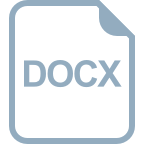