编写一个程序,用成员函数重载运算 符“+”和“”,实现两个二维数组相加和相减,要求第一个二维数组的值由构造函数设置另一个二维数组的值由键盘输入。
时间: 2024-04-30 09:18:32 浏览: 73
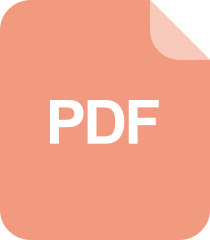
C++运算符重载 成员函数与友元函数详解

以下是一个示例程序,可以实现两个二维数组的相加和相减操作:
```cpp
#include <iostream>
using namespace std;
class Array2D {
private:
int rows, cols;
int** data;
public:
// 构造函数,设置第一个数组的值
Array2D(int r, int c) {
rows = r;
cols = c;
data = new int*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
for (int j = 0; j < cols; j++) {
data[i][j] = i + j; // 设置默认值
}
}
}
// 析构函数,释放内存
~Array2D() {
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
}
// 重载运算符 "+"
Array2D operator+(Array2D& other) {
if (rows != other.rows || cols != other.cols) {
cout << "Error: Arrays have different sizes." << endl;
return *this;
}
Array2D result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
// 重载运算符 "-"
Array2D operator-(Array2D& other) {
if (rows != other.rows || cols != other.cols) {
cout << "Error: Arrays have different sizes." << endl;
return *this;
}
Array2D result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] - other.data[i][j];
}
}
return result;
}
// 输入二维数组的值
void input() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cin >> data[i][j];
}
}
}
// 输出二维数组的值
void output() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
};
int main() {
// 创建两个二维数组
Array2D arr1(2, 3);
Array2D arr2(2, 3);
// 输入第二个二维数组的值
cout << "Please enter the values of the second array:" << endl;
arr2.input();
// 计算两个二维数组的和与差
Array2D sum = arr1 + arr2;
Array2D diff = arr1 - arr2;
// 输出结果
cout << "Array 1:" << endl;
arr1.output();
cout << "Array 2:" << endl;
arr2.output();
cout << "Sum:" << endl;
sum.output();
cout << "Difference:" << endl;
diff.output();
return 0;
}
```
上述程序中,`Array2D` 类表示一个二维数组,其中 `rows` 和 `cols` 分别表示数组的行数和列数,`data` 是一个二级指针,指向数组元素的内存空间。构造函数会为第一个数组设置默认值,析构函数会释放内存。`input` 和 `output` 函数分别用于输入和输出数组的值。`operator+` 和 `operator-` 函数分别重载了 "+" 和 "-" 运算符,可以实现两个二维数组的相加和相减操作。在主函数中,我们首先创建了两个二维数组 `arr1` 和 `arr2`,并通过 `input` 函数输入了 `arr2` 的值。然后,我们分别计算了 `arr1` 和 `arr2` 的和与差,最后通过 `output` 函数输出了结果。
阅读全文
相关推荐
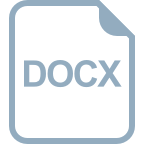
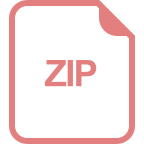















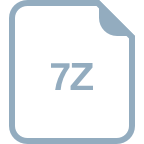