unity 自定义方法实现缓动方法代码实现
时间: 2023-12-09 20:03:13 浏览: 79
如果你想自定义一个缓动方法,可以使用委托和 Lambda 表达式来实现。下面是一个自定义的缓动方法示例:
```csharp
using UnityEngine;
using System;
public class CustomEasing : MonoBehaviour
{
private Vector3 start;
private Vector3 end;
private float duration;
private Action<Vector3> onValueChanged;
public void StartTween(Vector3 start, Vector3 end, float duration, Action<Vector3> onValueChanged)
{
this.start = start;
this.end = end;
this.duration = duration;
this.onValueChanged = onValueChanged;
StartCoroutine(Tween());
}
private IEnumerator Tween()
{
float t = 0f;
while (t < duration)
{
t += Time.deltaTime;
float p = t / duration;
Vector3 value = Vector3.Lerp(start, end, EasingFunction(p));
onValueChanged?.Invoke(value);
yield return null;
}
onValueChanged?.Invoke(end);
}
private Func<float, float> EasingFunction = p => p; // 默认为线性缓动
public void SetEasingFunction(Func<float, float> easingFunction)
{
EasingFunction = easingFunction;
}
}
```
上面的代码中,我们定义了一个 CustomEasing 类,它包含了一个 StartTween 方法和一个 SetEasingFunction 方法。StartTween 方法用于启动缓动,它需要传入起始值、结束值、缓动时间和回调函数,回调函数将在每一帧更新缓动值。SetEasingFunction 方法用于设置缓动函数,缓动函数是一个委托,它接受一个参数 t,表示缓动进度,返回一个缓动值。
在 StartTween 方法中,我们使用 StartCoroutine 方法启动了一个协程,在协程中不断更新缓动值,并在每一帧调用回调函数。缓动值的计算使用了 Mathf.Lerp 方法和缓动函数。最后,在缓动结束时,我们调用了回调函数,将最终值传递出去。
使用时,你可以先创建一个 CustomEasing 对象,然后使用 SetEasingFunction 方法设置缓动函数,最后使用 StartTween 方法启动缓动。例如,下面是一个使用弹性缓动的示例:
```csharp
CustomEasing easing = new CustomEasing();
easing.SetEasingFunction(p => Mathf.Sin(p * Mathf.PI * (0.2f + 2.5f * p * p * p)) * Mathf.Pow(1f - p, 2.2f) + p);
easing.StartTween(Vector3.zero, Vector3.one, 1f, value => transform.position = value);
```
在这个示例中,我们先创建了一个 CustomEasing 对象,并使用 SetEasingFunction 方法设置了弹性缓动函数。然后,我们调用 StartTween 方法启动缓动,将起始值、结束值、缓动时间和回调函数传递进去。回调函数中将更新物体的位置。
阅读全文
相关推荐
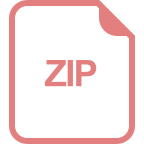
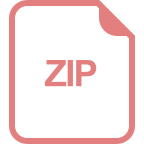
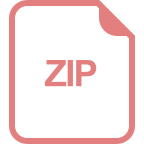
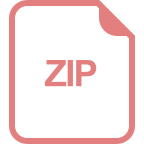
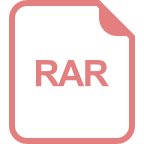
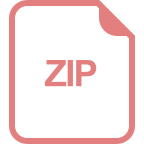
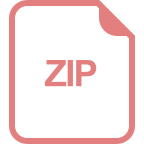
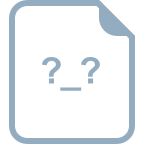
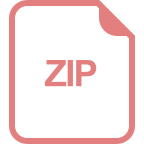
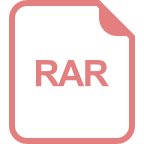
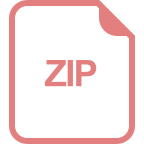
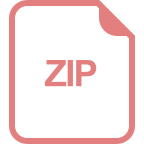
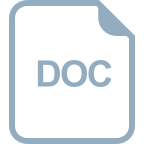
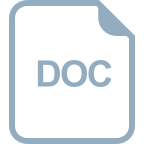
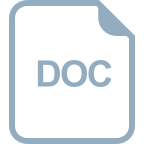
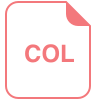
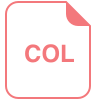
