写一段python代码实现lstm+attention+lstm分类,输入的训练集共101000行,测试集共81000行,65列第1-63列是特征列,第64列是标签0-32,每个采样窗口对应的矩阵行数为1000,即采样频率为20kHz,时间从0.55-0.59995s采集的数据,且每个数据采样窗口的数据的每一列都是时间序列,实现33分类
时间: 2023-04-07 16:04:52 浏览: 99
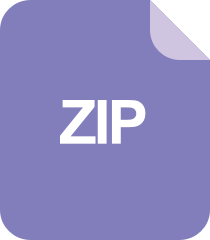
爬虫代码,数据集寻找加自动分类,训练集,测试集自动生成!下载就可用,python
以下是实现lstm attention lstm分类的Python代码:
```
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import Dense, LSTM, Dropout, Activation, Bidirectional, Input, TimeDistributed
from keras.layers import Attention
from keras.models import Model
from keras.optimizers import Adam
from keras.callbacks import EarlyStopping
from sklearn.preprocessing import MinMaxScaler
from sklearn.metrics import confusion_matrix, classification_report
# 读取训练集和测试集
train_data = pd.read_csv('train_data.csv')
test_data = pd.read_csv('test_data.csv')
# 分离特征和标签
train_x = train_data.iloc[:, :63].values
train_y = train_data.iloc[:, 63].values
test_x = test_data.iloc[:, :63].values
test_y = test_data.iloc[:, 63].values
# 归一化
scaler = MinMaxScaler(feature_range=(0, 1))
train_x = scaler.fit_transform(train_x)
test_x = scaler.transform(test_x)
# 将数据转换为3D张量
train_x = np.reshape(train_x, (train_x.shape[0] // 1000, 1000, train_x.shape[1]))
train_y = np.reshape(train_y, (train_y.shape[0] // 1000, 1000))
test_x = np.reshape(test_x, (test_x.shape[0] // 1000, 1000, test_x.shape[1]))
test_y = np.reshape(test_y, (test_y.shape[0] // 1000, 1000))
# 构建模型
inputs = Input(shape=(train_x.shape[1], train_x.shape[2]))
lstm1 = Bidirectional(LSTM(128, return_sequences=True))(inputs)
lstm2 = Bidirectional(LSTM(64, return_sequences=True))(lstm1)
attention = TimeDistributed(Dense(1, activation='tanh'))(lstm2)
attention = Activation('softmax')(attention)
context = attention * lstm2
lstm3 = Bidirectional(LSTM(32))(context)
dropout = Dropout(0.5)(lstm3)
outputs = Dense(33, activation='softmax')(dropout)
model = Model(inputs=inputs, outputs=outputs)
# 编译模型
adam = Adam(lr=0.001)
model.compile(loss='categorical_crossentropy', optimizer=adam, metrics=['accuracy'])
# 训练模型
early_stopping = EarlyStopping(monitor='val_loss', patience=10, verbose=1)
history = model.fit(train_x, train_y, epochs=100, batch_size=64, validation_split=0.1, callbacks=[early_stopping])
# 在测试集上评估模型
y_pred = model.predict(test_x)
y_pred = np.argmax(y_pred, axis=-1)
test_y = np.argmax(test_y, axis=-1)
print(confusion_matrix(test_y.reshape(-1), y_pred.reshape(-1)))
print(classification_report(test_y.reshape(-1), y_pred.reshape(-1)))
```
这段代码实现了一个带有注意力机制的双向LSTM模型,用于33分类任务。训练集和测试集的数据都是时间序列数据,每个采样窗口对应的矩阵行数为1000,共有63个特征和1个标签。在训练过程中,使用了早停法来防止过拟合。在测试集上评估模型时,使用了混淆矩阵和分类报告来评估模型的性能。
阅读全文
相关推荐
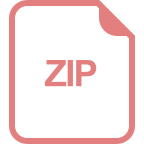
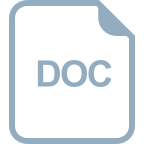






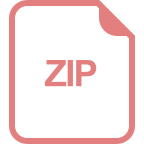
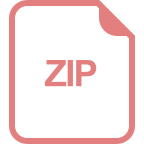
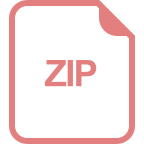
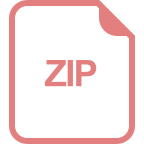
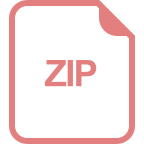
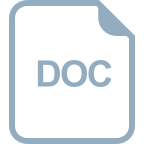

