bilstm+attention的torch代码
时间: 2023-09-03 09:12:11 浏览: 107
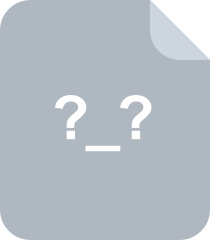
基于torch实现cnn+lstm+attention 模型时间序列预测 代码模板 通用

下面是一个基于 PyTorch 的 BiLSTM Attention 模型的示例代码:
```python
import torch
import torch.nn as nn
class BiLSTMAttention(nn.Module):
def __init__(self, input_size, hidden_size, num_classes):
super(BiLSTMAttention, self).__init__()
self.hidden_size = hidden_size
self.num_classes = num_classes
self.lstm = nn.LSTM(input_size, hidden_size, bidirectional=True, batch_first=True)
self.fc = nn.Linear(hidden_size*2, num_classes)
self.attention_layer = nn.Sequential(
nn.Linear(hidden_size*2, hidden_size),
nn.Tanh(),
nn.Linear(hidden_size, 1)
)
def forward(self, x):
lstm_out, _ = self.lstm(x)
attention_weights = self.attention_layer(lstm_out).squeeze(2)
attention_weights = torch.softmax(attention_weights, dim=1)
weighted_lstm_out = torch.bmm(lstm_out.permute(0,2,1), attention_weights.unsqueeze(2)).squeeze(2)
out = self.fc(weighted_lstm_out)
return out
```
在这个模型中,我们使用 `nn.LSTM` 来实现 BiLSTM,使用 `nn.Linear` 实现全连接层,使用 `nn.Sequential` 实现 Attention 层。
在 `forward` 方法中,我们首先使用 BiLSTM 对输入进行编码,然后通过 Attention 层计算每个时间步的注意力权重,将这些权重加权求和得到加权后的输出向量,最后通过全连接层输出分类结果。
这个模型的输入 `x` 是一个形状为 `(batch_size, seq_len, input_size)` 的张量,其中 `batch_size` 表示批次大小,`seq_len` 表示序列长度,`input_size` 表示输入特征维度。输出是一个形状为 `(batch_size, num_classes)` 的张量,其中 `num_classes` 表示分类类别数。
阅读全文
相关推荐
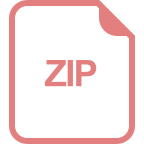
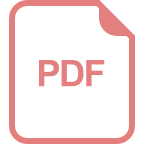














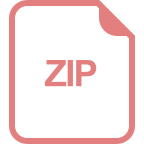
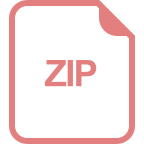