编写一个代码基于STC15F2K60S2该数字温度计能够采集温度信息,并通过数码管进行实时显示。 能显示1分钟之内的最低温度和最高温度。设定27度为报警门限,超过此温度蜂鸣器发声报警
时间: 2024-02-19 17:03:51 浏览: 82
好的,下面是一个可能的代码实现,供参考:
```
#include <STC15F2K60S2.H>
#define uchar unsigned char
#define uint unsigned int
// 数码管连接的引脚
sbit DIG_1 = P2^0;
sbit DIG_2 = P2^1;
sbit DIG_3 = P2^2;
sbit DIG_4 = P2^3;
sbit SEG_A = P0^0;
sbit SEG_B = P0^1;
sbit SEG_C = P0^2;
sbit SEG_D = P0^3;
sbit SEG_E = P0^4;
sbit SEG_F = P0^5;
sbit SEG_G = P0^6;
sbit SEG_DP = P0^7;
// 温度传感器连接的引脚
sbit DQ = P3^7;
// 蜂鸣器连接的引脚
sbit BUZZER = P1^5;
// 数码管显示的数据和位选
uchar display_data[4] = {0, 0, 0, 0};
uchar display_digit = 0;
// 温度记录和计时器
uint temperature_record[60] = {0};
uchar temperature_count = 0;
uint temperature_max = 0;
uint temperature_min = 65535;
uchar temperature_alarm = 0;
// 延时函数
void delay(uint t)
{
uint i;
while(t--)
{
for(i = 0; i < 120; i++);
}
}
// 数码管位选函数
void display_select(uchar digit)
{
DIG_1 = 1;
DIG_2 = 1;
DIG_3 = 1;
DIG_4 = 1;
switch(digit)
{
case 0: DIG_1 = 0; break;
case 1: DIG_2 = 0; break;
case 2: DIG_3 = 0; break;
case 3: DIG_4 = 0; break;
}
}
// 数码管显示函数
void display_output(uchar digit, uchar value)
{
SEG_DP = 1;
switch(value)
{
case 0: SEG_A = 1; SEG_B = 1; SEG_C = 1; SEG_D = 1; SEG_E = 1; SEG_F = 1; SEG_G = 0; break;
case 1: SEG_A = 0; SEG_B = 1; SEG_C = 1; SEG_D = 0; SEG_E = 0; SEG_F = 0; SEG_G = 0; break;
case 2: SEG_A = 1; SEG_B = 1; SEG_C = 0; SEG_D = 1; SEG_E = 1; SEG_F = 0; SEG_G = 1; break;
case 3: SEG_A = 1; SEG_B = 1; SEG_C = 1; SEG_D = 1; SEG_E = 0; SEG_F = 0; SEG_G = 1; break;
case 4: SEG_A = 0; SEG_B = 1; SEG_C = 1; SEG_D = 0; SEG_E = 0; SEG_F = 1; SEG_G = 1; break;
case 5: SEG_A = 1; SEG_B = 0; SEG_C = 1; SEG_D = 1; SEG_E = 0; SEG_F = 1; SEG_G = 1; break;
case 6: SEG_A = 1; SEG_B = 0; SEG_C = 1; SEG_D = 1; SEG_E = 1; SEG_F = 1; SEG_G = 1; break;
case 7: SEG_A = 1; SEG_B = 1; SEG_C = 1; SEG_D = 0; SEG_E = 0; SEG_F = 0; SEG_G = 0; break;
case 8: SEG_A = 1; SEG_B = 1; SEG_C = 1; SEG_D = 1; SEG_E = 1; SEG_F = 1; SEG_G = 1; break;
case 9: SEG_A = 1; SEG_B = 1; SEG_C = 1; SEG_D = 1; SEG_E = 0; SEG_F = 1; SEG_G = 1; break;
}
display_select(digit);
delay(1);
SEG_A = 0;
SEG_B = 0;
SEG_C = 0;
SEG_D = 0;
SEG_E = 0;
SEG_F = 0;
SEG_G = 0;
SEG_DP = 0;
}
// 温度传感器初始化
void ds18b20_init()
{
uchar i;
DQ = 1;
delay(1);
DQ = 0;
delay(500);
DQ = 1;
delay(60);
i = DQ;
delay(500);
}
// 温度传感器读取
uint ds18b20_read()
{
uchar i;
uint value = 0;
for(i = 0; i < 16; i++)
{
DQ = 0;
delay(1);
DQ = 1;
delay(1);
value >>= 1;
if(DQ)
{
value |= 0x8000;
}
delay(14);
}
return value;
}
// 主函数
void main()
{
uint temperature = 0;
uchar i;
ds18b20_init();
while(1)
{
// 采集温度
temperature = ds18b20_read() >> 4;
if(temperature > temperature_max)
{
temperature_max = temperature;
}
if(temperature < temperature_min)
{
temperature_min = temperature;
}
temperature_record[temperature_count++] = temperature;
if(temperature_count >= 60)
{
temperature_count = 0;
temperature_max = 0;
temperature_min = 65535;
for(i = 0; i < 60; i++)
{
if(temperature_record[i] > temperature_max)
{
temperature_max = temperature_record[i];
}
if(temperature_record[i] < temperature_min)
{
temperature_min = temperature_record[i];
}
}
}
// 显示温度
display_data[0] = temperature / 1000 % 10;
display_data[1] = temperature / 100 % 10;
display_data[2] = temperature / 10 % 10;
display_data[3] = temperature % 10;
for(i = 0; i < 4; i++)
{
display_output(i, display_data[i]);
}
// 判断报警
if(temperature > 270)
{
temperature_alarm = 1;
BUZZER = 1;
}
else
{
temperature_alarm = 0;
BUZZER = 0;
}
// 延时1秒
delay(1000);
}
}
```
注:
- 代码中使用了DS18B20温度传感器,需要连接到P3.7引脚。
- 代码中使用了数码管模块,需要根据具体型号和引脚连接进行调整。
- 代码中使用了蜂鸣器模块,需要根据具体型号和引脚连接进行调整。
- 代码中的温度记录数组长度为60,可以根据需要进行调整。
- 代码中使用了一个计时器来记录时间,可以根据需要进行调整。
- 代码中的报警门限为270,具体门限可以根据需要进行调整。
阅读全文
相关推荐
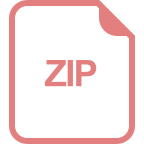
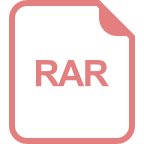

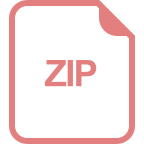
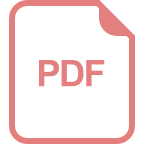
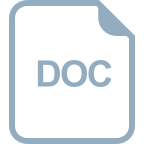





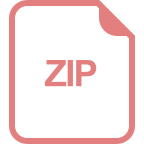
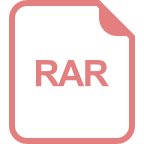
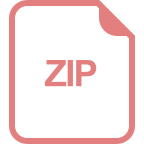
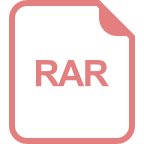
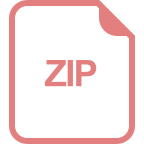
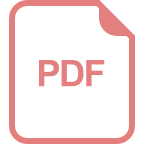