写一个基于 Python 和tkiner 的百度aip人体关键点识别GUI代码
时间: 2024-03-23 13:37:49 浏览: 23
好的,以下是一个基于 Python 和 Tkinter 的百度aip人体关键点识别GUI代码示例:
```python
import tkinter as tk
from tkinter import filedialog, messagebox
from PIL import Image, ImageTk
from aip import AipBodyAnalysis
# 设置百度aip的参数
APP_ID = 'your_app_id'
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
client = AipBodyAnalysis(APP_ID, API_KEY, SECRET_KEY)
class MainWindow:
def __init__(self, master):
# 创建主窗口
self.master = master
self.master.title('人体关键点识别')
self.master.geometry('800x600')
# 创建标签和按钮
self.label = tk.Label(self.master)
self.label.pack(side='left', padx=50, pady=50)
self.button = tk.Button(self.master, text='选择图片', command=self.select_image)
self.button.pack(side='right', padx=50, pady=50)
def select_image(self):
# 打开文件选择对话框
file_name = filedialog.askopenfilename(filetypes=[('Image files', '*.jpg;*.png')])
# 调用百度aip的人体关键点识别接口
with open(file_name, 'rb') as f:
image_data = f.read()
result = client.bodyAnalysis(image_data)
# 获取人体关键点坐标
body_parts = result['person_info'][0]['body_parts']
points = []
for part in body_parts.values():
x, y = part['x'], part['y']
points.append((x, y))
# 在图片上绘制关键点
image = Image.open(file_name)
draw = ImageDraw.Draw(image)
for point in points:
draw.ellipse((point[0]-5, point[1]-5, point[0]+5, point[1]+5), fill=(255, 0, 0))
image_tk = ImageTk.PhotoImage(image)
# 显示图片
self.label.configure(image=image_tk)
self.label.image = image_tk
if __name__ == '__main__':
# 创建应用程序
root = tk.Tk()
app = MainWindow(root)
root.mainloop()
```
在运行代码之前,需要先安装以下依赖库:
- Tkinter
- Pillow
- baidu-aip
你需要将 `your_app_id`、`your_api_key` 和 `your_secret_key` 替换为你自己的百度aip应用参数。运行程序后,点击按钮选择一张图片,程序会自动调用百度aip的人体关键点识别接口,获取关键点坐标,并在图片上绘制出关键点。
相关推荐
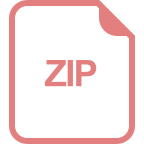
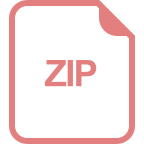
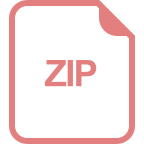














