定义接口或类 Shape,定义求周长的方法length()。 定义如下类,实现接口Shape或父类Shape的方法。 (1)三角形类Triangle (2)长方形类Rectangle (3)圆形类Circle等。 定义测试类ShapeTest,用Shape接口(或类)定义变量shape,用其指向不同类形的对象,输出各种图形的周长。并为其他的Shape接口实现类提供良好的扩展性。 提示: 计算圆周长时PI取3.14。 输入格式: 输入多组数值型数据(double); 一行中若有1个数,表示圆的半径; 一行中若有2个数(中间用空格间隔),表示长方形的长度、宽度。 一行中若有3个数(中间用空格间隔),表示三角形的三边的长度。(需要判断三个边长是否能构成三角形) 若输入数据中有0或负数,则不表示任何图形,周长为0。
时间: 2023-05-26 10:01:59 浏览: 76
Java代码如下:
接口Shape:
public interface Shape {
public abstract double length();
}
类Triangle:
public class Triangle implements Shape {
private double a;
private double b;
private double c;
public Triangle(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
public double length() {
if (a <= 0 || b <= 0 || c <= 0 || a+b <= c || a+c <= b || b+c <= a) {
return 0;
} else {
return a + b + c;
}
}
}
类Rectangle:
public class Rectangle implements Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double length() {
if (length <= 0 || width <= 0) {
return 0;
} else {
return 2 * (length + width);
}
}
}
类Circle:
public class Circle implements Shape {
private double radius;
private final double PI = 3.14;
public Circle(double radius) {
this.radius = radius;
}
public double length() {
if (radius <= 0) {
return 0;
} else {
return 2 * PI * radius;
}
}
}
测试类ShapeTest:
import java.util.Scanner;
public class ShapeTest {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Shape shape;
double[] params = new double[3];
while (scanner.hasNext()) {
int count = 0;
while (scanner.hasNextDouble() && count < 3) {
params[count++] = scanner.nextDouble();
}
if (count == 1) {
shape = new Circle(params[0]);
} else if (count == 2) {
shape = new Rectangle(params[0], params[1]);
} else if (count == 3) {
shape = new Triangle(params[0], params[1], params[2]);
} else {
break;
}
System.out.printf("%.2f\n", shape.length());
}
}
}
阅读全文
相关推荐
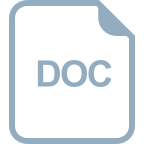
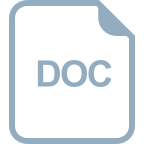
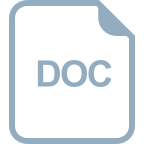












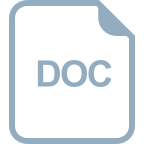
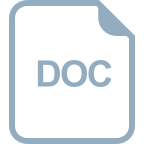
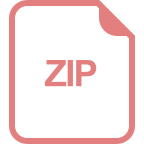
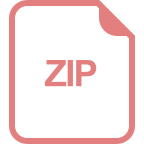