解释这段代码// 保存成绩表到文件void saveToFile() { ofstream file("students.txt"); if (!file.is_open()) { cout << "无法打开文件!\n"; return; } file << numStudents << "\n"; for (int i = 0; i < numStudents; i++) { file << students[i].id << " " << students[i].name << " "; for (int j = 0; j < NUM_SUBJECTS; j++) { file << students[i].scores[j] << " "; } file << students[i].averageScore << "\n"; } file.close(); cout << "保存成功!\n";}
时间: 2024-04-28 21:24:12 浏览: 9
这段代码是一个保存成绩表到文件的函数,其具体实现如下:
1. 首先创建一个 ofstream 对象,并传入文件名作为参数,打开文件用于写操作。
2. 判断文件是否成功打开,如果没有打开,则输出一条错误信息并直接返回。
3. 写入学生数量到文件的第一行。
4. 循环遍历每个学生,将学生的 id、name、scores 和 averageScore 写入文件中。
5. 关闭文件。
6. 输出保存成功的信息。
需要注意的是,这段代码中使用了一个常量 NUM_SUBJECTS,它代表每个学生的科目数量。同时,学生的信息包括 id、name、scores 和 averageScore,其中 scores 是一个数组,保存了每个学生的各科成绩。
相关问题
在Activity中,可以得到/data/data/com.itheima.savetofile/info.txt路径的输入流的方法是
在 `Activity` 中,可以通过 `openFileInput()` 方法得到 `/data/data/com.itheima.savetofile/info.txt` 路径的输入流,具体方法如下:
```java
try {
// 获取输入流
FileInputStream fis = openFileInput("info.txt");
// 读取数据
byte[] buffer = new byte[1024];
int len;
StringBuilder sb = new StringBuilder();
while ((len = fis.read(buffer)) != -1) {
sb.append(new String(buffer, 0, len));
}
// 关闭输入流
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
```
上述代码中,首先调用 `openFileInput()` 方法获取输入流,然后读取数据,最后关闭输入流。需要注意的是,如果文件不存在,会抛出 `FileNotFoundException` 异常。此外,如果需要将数据写入文件,可以使用 `openFileOutput()` 方法获取输出流。
void saveToFile() { FILE *file = fopen("student.txt", "w"); if (file == NULL) { printf("无法打开文件 student.txt。\n"); exit(1); } int i; for (i = 0; i < num_students; i++) { fprintf(file, "%d %s %s %d %d %d %d %d %d\n", students[i].num, students[i].name, students[i].sex, students[i].birthday_year, students[i].birthday_month, students[i].birthday_day, students[i].math_score, students[i].c_score, students[i].physics_score); } fclose(file); printf("学生成绩信息已保存到 student.txt。\n"); }修改并完善这段代码
此段代码是一个保存学生成绩信息到文件的函数,我们可以根据实际需求进行修改和完善。以下是可能需要的修改和完善:
1. 添加注释:在代码中添加注释,说明函数的作用、参数和返回值等信息,方便其他人理解和使用该函数。
2. 错误处理:在函数中添加错误处理代码,例如当文件无法打开时,输出错误信息并退出程序。
3. 文件路径:文件路径应该根据实际情况进行修改,避免出现无法打开文件的错误。
4. 文件格式:文件格式应该根据需求进行修改,例如可以使用逗号或制表符分隔数据,方便后续处理。
5. 代码风格:代码应该遵循一定的代码风格,例如统一缩进、命名规范等,方便代码阅读和维护。
修改后的代码如下:
```c
/**
* @brief 将学生成绩信息保存到文件中
*
* @param filename 文件名
* @param students 学生信息数组
* @param num_students 学生数量
* @return int 返回保存的学生数量
*/
int saveToFile(const char *filename, const student_t *students, int num_students) {
if (filename == NULL || students == NULL || num_students <= 0) {
return -1;
}
// 打开文件
FILE *file = fopen(filename, "w");
if (file == NULL) {
printf("无法打开文件 %s。\n", filename);
exit(1);
}
int i;
for (i = 0; i < num_students; i++) {
// 将学生信息写入文件
fprintf(file, "%d,%s,%s,%d,%d,%d,%d,%d,%d\n", students[i].num,
students[i].name, students[i].sex, students[i].birthday_year,
students[i].birthday_month, students[i].birthday_day,
students[i].math_score, students[i].c_score, students[i].physics_score);
}
// 关闭文件
fclose(file);
printf("学生成绩信息已保存到 %s。\n", filename);
return num_students;
}
```
相关推荐
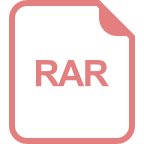
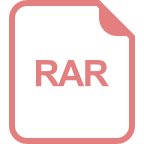
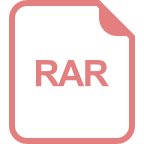












