用.NET语言写一个RSA加解密字符串的类,可以指定公钥和私钥
时间: 2024-02-22 18:57:53 浏览: 254
以下是基于.NET语言(C#)的RSA加解密字符串的类,可以指定公钥和私钥:
```csharp
using System;
using System.Security.Cryptography;
using System.Text;
public class RSAEncryptor
{
private RSAParameters publicKey;
private RSAParameters privateKey;
public RSAEncryptor(string publicKey, string privateKey)
{
RSACryptoServiceProvider rsa = new RSACryptoServiceProvider();
rsa.FromXmlString(publicKey);
this.publicKey = rsa.ExportParameters(false);
rsa = new RSACryptoServiceProvider();
rsa.FromXmlString(privateKey);
this.privateKey = rsa.ExportParameters(true);
}
public string Encrypt(string plainText)
{
byte[] plainBytes = Encoding.UTF8.GetBytes(plainText);
byte[] encryptedBytes;
using (RSACryptoServiceProvider rsa = new RSACryptoServiceProvider())
{
rsa.ImportParameters(publicKey);
encryptedBytes = rsa.Encrypt(plainBytes, true);
}
return Convert.ToBase64String(encryptedBytes);
}
public string Decrypt(string encryptedText)
{
byte[] encryptedBytes = Convert.FromBase64String(encryptedText);
byte[] decryptedBytes;
using (RSACryptoServiceProvider rsa = new RSACryptoServiceProvider())
{
rsa.ImportParameters(privateKey);
decryptedBytes = rsa.Decrypt(encryptedBytes, true);
}
return Encoding.UTF8.GetString(decryptedBytes);
}
}
```
使用示例:
```csharp
string publicKey = "<RSAKeyValue><Modulus>...</Modulus><Exponent>...</Exponent></RSAKeyValue>";
string privateKey = "<RSAKeyValue><Modulus>...</Modulus><Exponent>...</Exponent><P>...</P><Q>...</Q><DP>...</DP><DQ>...</DQ><InverseQ>...</InverseQ><D>...</D></RSAKeyValue>";
RSAEncryptor encryptor = new RSAEncryptor(publicKey, privateKey);
string plainText = "Hello, world!";
string encryptedText = encryptor.Encrypt(plainText);
string decryptedText = encryptor.Decrypt(encryptedText);
Console.WriteLine(encryptedText);
Console.WriteLine(decryptedText);
```
请注意:在使用时,应该将实际的公钥和私钥替换为实际的值。此处给出的是XML格式的RSA密钥对,也可以使用其他格式的密钥对。
阅读全文
相关推荐
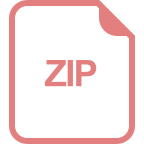
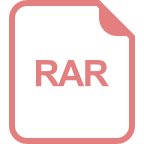
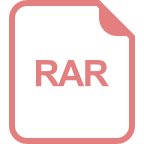















