rsa生成公钥和私钥加解密生成公钥私钥c语言
时间: 2024-06-18 16:05:00 浏览: 17
RSA是一种非对称加密算法,其公钥和私钥是成对生成的。以下是RSA生成公钥和私钥以及加解密的步骤:
1. 生成RSA公钥和私钥:
首先需要随机生成两个大素数p和q,计算n = p * q,再选取一个整数e(一般为65537),计算d = e^-1 mod ((p-1) * (q-1))。
生成的公钥为(n, e),私钥为(n, d)。
2. RSA加密:
假设要将明文M加密为密文C,使用公钥(n, e)进行加密,计算C = M^e mod n。
3. RSA解密:
使用私钥(n, d)进行解密,计算M = C^d mod n。
以下是一个简单的RSA加解密的C语言实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/bio.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
int main()
{
// 生成RSA公钥和私钥
RSA *rsa = RSA_generate_key(2048, 65537, NULL, NULL);
if (rsa == NULL) {
printf("Failed to generate RSA key pair.\n");
return -1;
}
// 获取公钥和私钥
BIO *pubBio = BIO_new(BIO_s_mem());
BIO *priBio = BIO_new(BIO_s_mem());
PEM_write_bio_RSAPublicKey(pubBio, rsa);
PEM_write_bio_RSAPrivateKey(priBio, rsa, NULL, NULL, 0, NULL, NULL);
// 获取公钥和私钥字符串
char pubKey[1024] = {0};
char priKey[4096] = {0};
BIO_read(pubBio, pubKey, sizeof(pubKey));
BIO_read(priBio, priKey, sizeof(priKey));
// 输出公钥和私钥
printf("Public Key:\n%s\n", pubKey);
printf("Private Key:\n%s\n", priKey);
// 加密明文
char *plaintext = "Hello World!";
int plaintextLen = strlen(plaintext) + 1;
char ciphertext[4096] = {0};
int ciphertextLen = RSA_public_encrypt(plaintextLen, (unsigned char *)plaintext,
(unsigned char *)ciphertext, rsa, RSA_PKCS1_PADDING);
if (ciphertextLen == -1) {
printf("Failed to encrypt plaintext.\n");
return -1;
}
// 输出密文
printf("Ciphertext:\n");
for (int i = 0; i < ciphertextLen; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// 解密密文
char decrypted[4096] = {0};
int decryptedLen = RSA_private_decrypt(ciphertextLen, (unsigned char *)ciphertext,
(unsigned char *)decrypted, rsa, RSA_PKCS1_PADDING);
if (decryptedLen == -1) {
printf("Failed to decrypt ciphertext.\n");
return -1;
}
// 输出解密后的明文
printf("Decrypted plaintext: %s\n", decrypted);
// 释放资源
RSA_free(rsa);
BIO_free_all(pubBio);
BIO_free_all(priBio);
return 0;
}
```
相关推荐
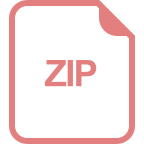














