用c语言写出公钥和私钥生成的函数
时间: 2024-12-24 20:04:52 浏览: 3
在C语言中生成公钥和私钥通常使用OpenSSL库。以下是一个示例代码,展示了如何使用OpenSSL库生成RSA公钥和私钥:
```c
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
#include <stdio.h>
#include <string.h>
void handleErrors(void)
{
ERR_print_errors_fp(stderr);
abort();
}
int generateRSAKeyPair(unsigned int keyLength, FILE **publicKeyFile, FILE **privateKeyFile)
{
RSA *rsa = NULL;
BIGNUM *bn = NULL;
BIO *bp_public = NULL, *bp_private = NULL;
int ret = 0;
// Initialize the OpenSSL library
OpenSSL_add_all_algorithms();
ERR_load_BIO_strings();
ERR_load_crypto_strings();
// Create a new RSA key
rsa = RSA_new();
if (rsa == NULL)
handleErrors();
bn = BN_new();
if (bn == NULL)
handleErrors();
// Generate a random number
ret = BN_set_word(bn, RSA_F4);
if (ret != 1)
handleErrors();
// Generate the RSA key
ret = RSA_generate_key_ex(rsa, keyLength, bn, NULL);
if (ret != 1)
handleErrors();
// Write the public key to a file
bp_public = BIO_new_file(publicKeyFile[0], "w+");
if (bp_public == NULL)
handleErrors();
ret = PEM_write_bio_RSAPublicKey(bp_public, rsa);
if (ret != 1)
handleErrors();
// Write the private key to a file
bp_private = BIO_new_file(privateKeyFile[0], "w+");
if (bp_private == NULL)
handleErrors();
ret = PEM_write_bio_RSAPrivateKey(bp_private, rsa, NULL, NULL, 0, NULL, NULL);
if (ret != 1)
handleErrors();
// Clean up
BIO_free(bp_public);
BIO_free(bp_private);
RSA_free(rsa);
BN_free(bn);
return 1;
}
int main()
{
FILE *publicKeyFile = fopen("public_key.pem", "w");
FILE *privateKeyFile = fopen("private_key.pem", "w");
if (publicKeyFile == NULL || privateKeyFile == NULL) {
printf("Error opening files for writing.\n");
return 1;
}
if (generateRSAKeyPair(2048, &publicKeyFile, &privateKeyFile)) {
printf("RSA key pair generated successfully.\n");
} else {
printf("Error generating RSA key pair.\n");
}
fclose(publicKeyFile);
fclose(privateKeyFile);
return 0;
}
```
这个示例代码使用了OpenSSL库来生成RSA公钥和私钥。你需要确保你的系统中已经安装了OpenSSL库,并在编译时链接OpenSSL库。例如:
```sh
gcc -o generate_keys generate_keys.c -lssl -lcrypto
```
阅读全文
相关推荐
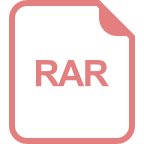
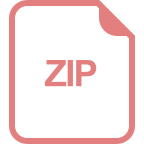
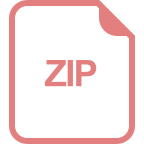


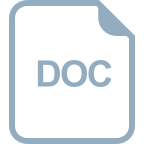
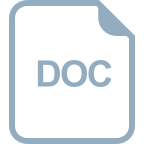
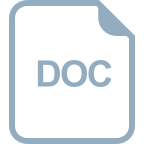











