STM32F103VET6基于Keil编写对DHT11温湿度传感器的控制代码
时间: 2023-07-26 11:28:16 浏览: 137
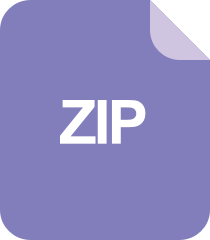
STM32控制DHT11数字温湿度传感器_DHT11_STM32F103_

好的,我可以为您提供基于Keil编写的STM32F103VET6对DHT11温湿度传感器的控制代码,如下所示:
```c
#include "stm32f10x.h"
#include "delay.h"
#define DHT11_GPIO GPIOB
#define DHT11_PIN GPIO_Pin_0
void DHT11_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_GPIO, &GPIO_InitStructure);
GPIO_SetBits(DHT11_GPIO, DHT11_PIN);
}
void DHT11_Start(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_GPIO, &GPIO_InitStructure);
GPIO_ResetBits(DHT11_GPIO, DHT11_PIN);
delay_ms(18);
GPIO_SetBits(DHT11_GPIO, DHT11_PIN);
delay_us(30);
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_GPIO, &GPIO_InitStructure);
}
u8 DHT11_Response(void)
{
u8 cnt = 0;
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_PIN) && cnt < 100)
{
cnt++;
delay_us(1);
}
if (cnt >= 100)
return 1;
cnt = 0;
while (!GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_PIN) && cnt < 100)
{
cnt++;
delay_us(1);
}
if (cnt >= 100)
return 1;
cnt = 0;
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_PIN) && cnt < 100)
{
cnt++;
delay_us(1);
}
if (cnt >= 100)
return 1;
return 0;
}
u8 DHT11_Read_Bit(void)
{
u8 cnt = 0;
while (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_PIN) && cnt < 100)
{
cnt++;
delay_us(1);
}
if (cnt >= 100)
return 1;
delay_us(40);
if (GPIO_ReadInputDataBit(DHT11_GPIO, DHT11_PIN))
return 1;
else
return 0;
}
u8 DHT11_Read_Byte(void)
{
u8 i, dat = 0;
for (i = 0; i < 8; i++)
{
dat <<= 1;
dat |= DHT11_Read_Bit();
}
return dat;
}
u8 DHT11_Read_Data(u8 *temperature, u8 *humidity)
{
u8 buf[5];
u8 i;
DHT11_Start();
if (DHT11_Response())
return 1;
for (i = 0; i < 5; i++)
buf[i] = DHT11_Read_Byte();
if ((buf[0] + buf[1] + buf[2] + buf[3]) == buf[4])
{
*humidity = buf[0];
*temperature = buf[2];
return 0;
}
else
return 1;
}
```
在以上代码中,使用了delay.h库提供的延时函数来实现精确的时间控制,需要注意的是,需要先在工程中添加delay.h和delay.c文件。
另外,在使用DHT11_Read_Data函数读取温湿度数据时,需要传入温度和湿度变量的指针,以便在函数内部进行数据的存储。
希望这份代码对您有所帮助!
阅读全文
相关推荐
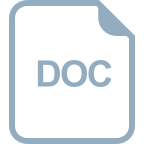

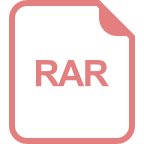
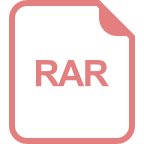
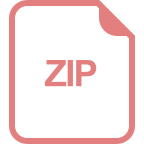
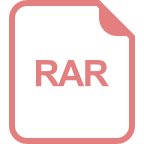
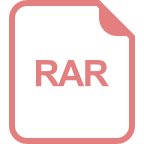
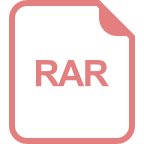
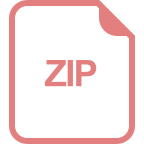
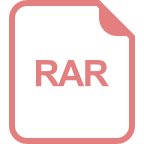
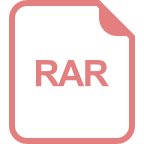

