MFC 将一张24位图转换成单色位图代码
时间: 2023-09-14 14:05:46 浏览: 48
### 回答1:
以下是 MFC 将一张 24 位图转换成单色位图的代码,其中使用了 GDI+ 库:
1. 首先需要添加头文件:
```cpp
#include <afxwin.h>
#include <afxwinapp.h>
#include <afxwin.h>
#include <afxext.h>
#include <afxdisp.h>
#include <afxdtctl.h>
#include <afxcmn.h>
#include <afxcontrolbars.h>
#include <gdiplus.h>
using namespace Gdiplus;
```
2. 然后定义一个函数来进行转换:
```cpp
void ConvertToMonoBitmap(CBitmap& bmpSrc, CBitmap& bmpDest)
{
// 获取源图像的宽度和高度
BITMAP bmpInfo;
bmpSrc.GetBitmap(&bmpInfo);
int width = bmpInfo.bmWidth;
int height = bmpInfo.bmHeight;
// 创建 GDI+ Bitmap 对象
Bitmap* pBitmap = Bitmap::FromHBITMAP(bmpSrc, NULL);
// 创建 GDI+ Graphics 对象
Graphics* pGraphics = Graphics::FromImage(pBitmap);
// 创建 GDI+ Bitmap 对象,用于存储转换后的图像
Bitmap* pMonoBitmap = new Bitmap(width, height);
// 创建 GDI+ Graphics 对象,用于绘制转换后的图像
Graphics* pMonoGraphics = Graphics::FromImage(pMonoBitmap);
// 创建 GDI+ SolidBrush 对象,用于绘制黑色和白色
SolidBrush brushBlack(Color(0, 0, 0));
SolidBrush brushWhite(Color(255, 255, 255));
// 遍历源图像的每个像素
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
// 获取源图像该像素的颜色值
Color color;
pBitmap->GetPixel(x, y, &color);
// 计算灰度值
int gray = (color.GetR() + color.GetG() + color.GetB()) / 3;
// 绘制单色位图
if (gray > 128)
pMonoGraphics->FillRectangle(&brushWhite, x, y, 1, 1);
else
pMonoGraphics->FillRectangle(&brushBlack, x, y, 1, 1);
}
}
// 将转换后的图像保存到目标位图中
bmpDest.Attach((HBITMAP)pMonoBitmap->GetHBITMAP(Color(), NULL));
// 释放资源
delete pBitmap;
delete pGraphics;
delete pMonoBitmap;
delete pMonoGraphics;
}
```
3. 在需要进行转换的地方调用该函数即可:
```cpp
// 加载源图像
CBitmap bmpSrc;
bmpSrc.LoadBitmap(IDB_BITMAP_SRC);
// 创建目标位图
CBitmap bmpDest;
bmpDest.CreateBitmap(100, 100, 1, 1, NULL);
// 转换图像
ConvertToMonoBitmap(bmpSrc, bmpDest);
// 显示目标图像
CStatic* pStatic = (CStatic*)GetDlgItem(IDC_STATIC_DEST);
pStatic->SetBitmap(bmpDest);
```
### 回答2:
在 MFC 中将一张24位图转换成单色位图的代码如下:
```cpp
CImage sourceImage;
CImage targetImage;
// 1. 加载原始图像
sourceImage.Load(_T("source.bmp"));
// 2. 获取原始图像的宽度和高度
int width = sourceImage.GetWidth();
int height = sourceImage.GetHeight();
// 3. 创建目标图像,与原始图像大小相同,像素格式为单色位图
targetImage.Create(width, height, 1);
// 4. 遍历原始图像的每个像素
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
// 5. 获取原始图像当前像素的 RGB 值
COLORREF rgb = sourceImage.GetPixel(x, y);
BYTE red = GetRValue(rgb);
BYTE green = GetGValue(rgb);
BYTE blue = GetBValue(rgb);
// 6. 根据灰度值计算目标图像当前像素的亮度值
BYTE brightness = static_cast<BYTE>(0.299 * red + 0.587 * green + 0.114 * blue);
// 7. 将亮度值设置为目标图像当前像素的颜色
targetImage.SetPixel(x, y, RGB(brightness, brightness, brightness));
}
}
// 8. 保存目标图像为单色位图
targetImage.Save(_T("target.bmp"));
```
以上代码首先加载一张24位的原始图像,然后创建一个与原始图像大小相同的单色位图。然后遍历原始图像的每个像素,获取其 RGB 值,然后根据灰度公式计算目标图像当前像素的亮度值。最后将亮度值设置为目标图像当前像素的颜色。最终,将目标图像保存为单色位图。
### 回答3:
要将一张24位图转换成单色位图,可以使用以下代码:
首先,我们需要创建一个CImage对象,并加载原始的24位图像文件:
CImage originalImage;
originalImage.Load(_T("原始图像路径"));
接下来,我们创建一个与原始图像大小相同的单色位图对象,并设置其宽度、高度以及像素格式:
CImage monoImage;
monoImage.Create(originalImage.GetWidth(), originalImage.GetHeight(), originalImage.GetBPP());
然后,我们需要遍历每个像素,将其转换为单色位图的像素值。对于24位图像,每个像素由红、绿、蓝三个颜色分量组成,我们可以使用GetPixel和SetPixel函数分别获取和设置像素值:
for (int y = 0; y < originalImage.GetHeight(); y++)
{
for (int x = 0; x < originalImage.GetWidth(); x++)
{
COLORREF color = originalImage.GetPixel(x, y);
// 将彩色像素转换为灰度值
int grayValue = (GetRValue(color) + GetGValue(color) + GetBValue(color)) / 3;
// 设置单色位图像素值(黑白二值化)
if (grayValue > 128)
monoImage.SetPixel(x, y, RGB(255, 255, 255)); // 白色
else
monoImage.SetPixel(x, y, RGB(0, 0, 0)); // 黑色
}
}
最后,我们保存转换后的单色位图到指定文件路径,并释放原始图像资源:
monoImage.Save(_T("单色位图路径"));
originalImage.Destroy();
以上是将一张24位图转换成单色位图的代码。其中,通过对彩色像素进行灰度化处理,并根据阈值将像素值设为黑色或白色,实现了单色位图的生成。
相关推荐
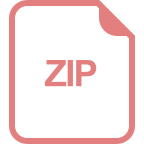










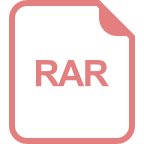
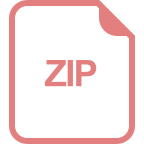