WARNING:tensorflow:Calling GradientTape.gradient on a persistent tape inside its context is significantly less efficient than calling it outside the context (it causes the gradient ops to be recorded on the tape, leading to increased CPU and memory usage). Only call GradientTape.gradient inside the context if you actually want to trace the gradient in order to compute higher order derivatives.
时间: 2024-02-07 10:02:23 浏览: 281
这个警告是因为当您在`tf.GradientTape`中调用`persistent=True`时,在上下文内调用`gradient()`函数会导致梯度操作被记录在磁带上,从而导致CPU和内存的大量使用。只有在需要计算更高阶导数时,才在上下文内调用`gradient()`函数以跟踪梯度。如果您只是需要计算一阶导数,请在上下文外调用`gradient()`函数,以避免这个警告和潜在的性能下降。例如,您可以这样写:
```
with tf.GradientTape(persistent=True) as tape:
# 计算损失函数
grads = tape.gradient(loss, model.trainable_variables)
del tape # 手动删除磁带
```
在上面的代码中,我们在上下文内计算了损失函数,但在上下文外调用了`gradient()`函数来计算梯度,并手动删除了磁带,以避免梯度操作被记录在磁带上。这样可以避免警告和潜在的性能下降。
相关问题
WARNING:tensorflow:From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\bert_base\bert\modeling.py:359: calling dropout (from tensorflow.python.ops.nn_ops) with keep_prob is deprecated and will be removed in a future version. Instructions for updating: Please use rate instead of keep_prob. Rate should be set to rate = 1 - keep_prob. WARNING:tensorflow:From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\bert_base\bert\modeling.py:673: dense (from tensorflow.python.layers.core) is deprecated and will be removed in a future version. Instructions for updating: Use keras.layers.Dense instead. WARNING:tensorflow:From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\venv\lib\site-packages\tensorflow_core\python\layers\core.py:187: Layer.apply (from tensorflow.python.keras.engine.base_layer) is deprecated and will be removed in a future version. Instructions for updating: Please use layer.__call__ method instead. WARNING:tensorflow:From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\bert_base\bert\modeling.py:277: The name tf.erf is deprecated. Please use tf.math.erf instead.
这些警告信息是关于一些 TensorFlow 函数和类的变化和更新的提示。我会逐个解释每个警告的含义:
1. "From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\bert_base\bert\modeling.py:359: calling dropout (from tensorflow.python.ops.nn_ops) with keep_prob is deprecated and will be removed in a future version. Instructions for updating: Please use rate instead of keep_prob. Rate should be set to rate = 1 - keep_prob."
这个警告表示在 "modeling.py" 文件的第 359 行中,使用了 `dropout` 函数,并传递了 `keep_prob` 参数。然而,将来的 TensorFlow 版本将移除 `keep_prob` 参数,并建议使用 `rate` 参数代替。`rate` 参数的计算方式为 `rate = 1 - keep_prob`。
你可以将代码中的 `keep_prob` 替换为 `rate` 来消除这个警告。
2. "From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\bert_base\bert\modeling.py:673: dense (from tensorflow.python.layers.core) is deprecated and will be removed in a future version. Instructions for updating: Use keras.layers.Dense instead."
这个警告表示在 "modeling.py" 文件的第 673 行中,使用了 `dense` 函数,但该函数已被废弃,并将在将来的 TensorFlow 版本中移除。建议使用 `keras.layers.Dense` 来代替。
你可以将代码中的 `dense` 替换为 `keras.layers.Dense` 来消除这个警告。
3. "From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\venv\lib\site-packages\tensorflow_core\python\layers\core.py:187: Layer.apply (from tensorflow.python.keras.engine.base_layer) is deprecated and will be removed in a future version. Instructions for updating: Please use layer.__call__ method instead."
这个警告表示在 "core.py" 文件的第 187 行中,使用了 `Layer.apply` 方法,但该方法已被废弃,并将在将来的 TensorFlow 版本中移除。建议使用 `layer.__call__` 方法来代替。
你可以将代码中的 `Layer.apply` 替换为 `layer.__call__` 来消除这个警告。
4. "From E:\pycharm-workspace\BERT\BERT-BiLSTM-CRF-NER-master\bert_base\bert\modeling.py:277: The name tf.erf is deprecated. Please use tf.math.erf instead."
这个警告表示在 "modeling.py" 文件的第 277 行中,使用了 `tf.erf` 函数,但该函数已被废弃。建议使用 `tf.math.erf` 函数来代替。
你可以将代码中的 `tf.erf` 替换为 `tf.math.erf` 来消除这个警告。
总之,这些警告信息是为了提醒你在代码中使用了已被废弃或即将被移除的 TensorFlow 函数和类。建议根据警告信息中的建议进行相应的更新,以保持代码的兼容性和稳定性。
如果你对这些警告信息有更多疑问或需要进一步帮助,请随时提问。
WARNING:tensorflow:From D:\PSMNet-TensorFlow-master\models\model_utils.py:66: calling softmax (from tensorflow.python.ops.nn_ops) with dim is deprecated and will be removed in a future version. Instructions for updating: dim is deprecated, use axis instead filtered_cost_volume: (8, 128, 256, 512) probability_volume: (8, 128, 256, 512)
这个警告是因为在 TensorFlow 2.0 中,`dim` 参数已被弃用,应该使用 `axis` 参数代替。因此,你需要将代码中所有使用 `dim` 参数的函数都修改为使用 `axis` 参数。例如,将:
```
import tensorflow as tf
x = tf.nn.softmax(logits, dim=1)
```
改为:
```
import tensorflow as tf
x = tf.nn.softmax(logits, axis=1)
```
这样就不会再出现这个警告了。
如果你不想看到这个警告,可以添加以下代码:
```
import logging
logging.getLogger('tensorflow').disabled = True
```
这将禁用 TensorFlow 的所有警告和信息输出。不过,这可能会使你在调试代码时失去一些有用的信息。
阅读全文
相关推荐
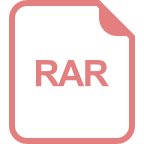
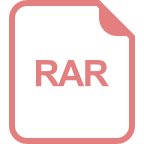
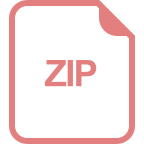













