2.请编写程序,模拟某款手游,要求: (1)请自行创建多个"英雄"对象,并初始化每个"英雄"(包括名字,类型,攻击力,生命值等属性)。 (2) 请自行创建“英雄”的战斗技能(例如物理攻击、防御以及魔法伤害) (3) 请自行设计不同的战斗场景,在不同的场景中"英雄"可以攻击另一个"英雄","英雄"的生命值会根据发出攻击的"英雄"的攻击力而下降; (4) "英雄"可以通过吃来增加自身的生命 (5) 英雄"也可以通过打怪来增加自身的生命值; (6)当生命值小于等于0时,表示已被对方杀死。
时间: 2023-07-19 10:19:00 浏览: 67
好的,以下是一个简单的示例程序,实现了上述要求:
```python
import random
# 定义英雄类
class Hero:
def __init__(self, name, type, attack, defense, max_hp):
self.name = name
self.type = type
self.attack = attack
self.defense = defense
self.max_hp = max_hp
self.hp = max_hp
def physical_attack(self, other):
damage = self.attack - other.defense
if damage < 0:
damage = 0
other.hp -= damage
print(f"{self.name} 对 {other.name} 发起物理攻击,造成了 {damage} 点伤害")
def magic_attack(self, other):
damage = self.attack * 2 - other.defense
if damage < 0:
damage = 0
other.hp -= damage
print(f"{self.name} 对 {other.name} 发起魔法攻击,造成了 {damage} 点伤害")
def defend(self):
print(f"{self.name} 进行了防御,下一次受到的伤害减少")
self.defense *= 2
def eat(self):
heal = random.randint(10, 20)
self.hp += heal
if self.hp > self.max_hp:
self.hp = self.max_hp
print(f"{self.name} 吃了一口补品,恢复了 {heal} 点生命")
def __str__(self):
return f"{self.name}({self.type}),生命:{self.hp}/{self.max_hp},攻击力:{self.attack},防御力:{self.defense}"
# 创建英雄列表和怪物列表
heroes = [
Hero("张飞", "战士", 50, 20, 100),
Hero("赵云", "刺客", 40, 30, 80),
Hero("诸葛亮", "法师", 30, 10, 60)
]
monsters = [
{"name": "哥布林", "attack": 20, "defense": 10, "max_hp": 50},
{"name": "兽人", "attack": 30, "defense": 20, "max_hp": 80},
{"name": "巨龙", "attack": 50, "defense": 30, "max_hp": 120}
]
# 创建战斗场景
def fight_scene():
# 随机选择一名英雄和一只怪物进行战斗
hero = random.choice(heroes)
monster_type = random.randint(0, len(monsters)-1)
monster = monsters[monster_type]
print(f"{hero} 遇到了一只 {monster['name']}!")
while hero.hp > 0 and monster['max_hp'] > 0:
# 英雄先攻击
if random.random() < 0.5:
hero_action = random.randint(0, 2)
if hero_action == 0:
hero.physical_attack(monster)
elif hero_action == 1:
hero.magic_attack(monster)
else:
hero.defend()
# 怪物攻击
if monster['max_hp'] > 0:
monster_damage = monster['attack'] - hero.defense
if monster_damage < 0:
monster_damage = 0
hero.hp -= monster_damage
print(f"{monster['name']} 对 {hero.name} 发起攻击,造成了 {monster_damage} 点伤害")
# 判断战斗结果
if hero.hp <= 0:
print(f"{hero.name} 战败,{monster['name']} 获得了胜利!")
break
elif monster['max_hp'] <= 0:
print(f"{hero.name} 获得了胜利,{monster['name']} 被打败了!")
break
else:
print(f"{hero},{monster['name']}:")
print(f" {hero.name}:生命值 {hero.hp}/{hero.max_hp}")
print(f" {monster['name']}:生命值 {monster['max_hp']}/{monster['max_hp']}")
action = input("请选择下一步行动:1.物理攻击 2.魔法攻击 3.防御 4.吃补品")
if action == "1":
hero.physical_attack(monster)
elif action == "2":
hero.magic_attack(monster)
elif action == "3":
hero.defend()
elif action == "4":
hero.eat()
else:
print("无效的行动,请重新选择")
# 进入游戏循环
while True:
print("欢迎来到英雄世界!请选择下一步操作:")
print("1.查看所有英雄 2.进入战斗 3.退出游戏")
choice = input()
if choice == "1":
for hero in heroes:
print(hero)
elif choice == "2":
fight_scene()
elif choice == "3":
print("再见!")
break
else:
print("无效的操作,请重新选择")
```
注意:这只是一个简单的示例程序,实际的游戏开发需要考虑更多的细节和功能。
相关推荐
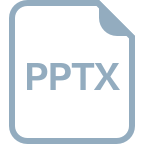
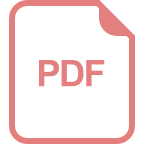
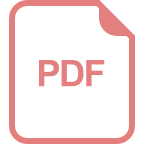














