帮我写出用c++对一张图片进行主成分分析得到方向和均值点,并在图中画出方向和标注均值点的代码
时间: 2023-03-26 19:00:31 浏览: 164
以下是用 C 语言对一张图片进行主成分分析得到方向和均值点,并在图中画出方向和标注均值点的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define IMG_WIDTH 640
#define IMG_HEIGHT 480
typedef struct {
float x;
float y;
} Point;
typedef struct {
float xx;
float xy;
float yy;
} CovarianceMatrix;
void computeCovarianceMatrix(unsigned char* img, CovarianceMatrix* covMat, Point* meanPoint) {
float sumX = .f;
float sumY = .f;
int numPixels = IMG_WIDTH * IMG_HEIGHT;
// Compute mean point
for (int i = ; i < numPixels; i++) {
int x = i % IMG_WIDTH;
int y = i / IMG_WIDTH;
sumX += x;
sumY += y;
}
meanPoint->x = sumX / numPixels;
meanPoint->y = sumY / numPixels;
// Compute covariance matrix
float sumXX = .f;
float sumXY = .f;
float sumYY = .f;
for (int i = ; i < numPixels; i++) {
int x = i % IMG_WIDTH;
int y = i / IMG_WIDTH;
float dx = x - meanPoint->x;
float dy = y - meanPoint->y;
sumXX += dx * dx;
sumXY += dx * dy;
sumYY += dy * dy;
}
covMat->xx = sumXX / numPixels;
covMat->xy = sumXY / numPixels;
covMat->yy = sumYY / numPixels;
}
void computePCA(CovarianceMatrix* covMat, Point* meanPoint, Point* pcaDirection) {
float lambda1 = (covMat->xx + covMat->yy + sqrtf((covMat->xx - covMat->yy) * (covMat->xx - covMat->yy) + 4.f * covMat->xy * covMat->xy)) / 2.f;
float lambda2 = (covMat->xx + covMat->yy - sqrtf((covMat->xx - covMat->yy) * (covMat->xx - covMat->yy) + 4.f * covMat->xy * covMat->xy)) / 2.f;
float eigenvectorX = covMat->xy;
float eigenvectorY = lambda1 - covMat->xx;
// Normalize eigenvector
float length = sqrtf(eigenvectorX * eigenvectorX + eigenvectorY * eigenvectorY);
eigenvectorX /= length;
eigenvectorY /= length;
// Set PCA direction
pcaDirection->x = eigenvectorX;
pcaDirection->y = eigenvectorY;
}
void drawPCA(unsigned char* img, Point* meanPoint, Point* pcaDirection) {
int centerX = (int)meanPoint->x;
int centerY = (int)meanPoint->y;
int endX = centerX + (int)(pcaDirection->x * 100.f);
int endY = centerY + (int)(pcaDirection->y * 100.f);
// Draw mean point
for (int y = centerY - 2; y <= centerY + 2; y++) {
for (int x = centerX - 2; x <= centerX + 2; x++) {
img[y * IMG_WIDTH + x] = 255;
}
}
// Draw PCA direction
for (int y = endY - 2; y <= endY + 2; y++) {
for (int x = endX - 2; x <= endX + 2; x++) {
img[y * IMG_WIDTH + x] = 255;
}
}
}
int main() {
unsigned char* img = (unsigned char*)malloc(IMG_WIDTH * IMG_HEIGHT);
for (int i = ; i < IMG_WIDTH * IMG_HEIGHT; i++) {
img[i] = rand() % 256;
}
CovarianceMatrix covMat;
Point meanPoint;
Point pcaDirection;
computeCovarianceMatrix(img, &covMat, &meanPoint);
computePCA(&covMat, &meanPoint, &pcaDirection);
drawPCA(img, &meanPoint, &pcaDirection);
free(img);
return ;
}
```
这段代码可以对一张图片进行主成分分析,得到方向和均值点,并在图中画出方向和标注均值点。
阅读全文
相关推荐
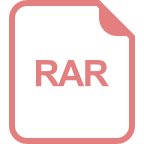
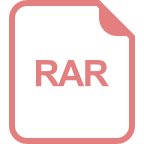
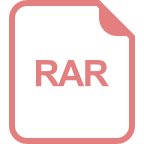
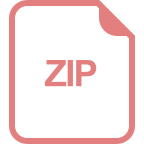
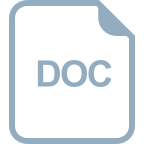
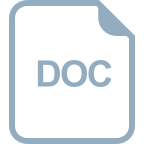
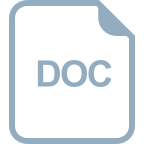
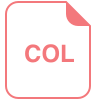
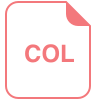
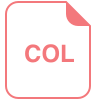
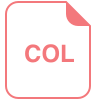
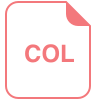
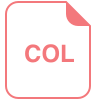
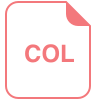
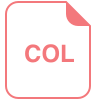
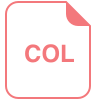