c++,PostMessage中的LPARAM的字符串怎么转换成qstring
时间: 2024-02-17 17:03:26 浏览: 24
在 Windows 中,`PostMessage` 函数可以使用 `LPARAM` 参数来传递字符串等数据。如果需要将 `LPARAM` 参数中的字符串转换为 `QString`,可以使用以下代码:
```cpp
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_MY_MESSAGE:
{
// 将 LPARAM 参数转换为字符串
const char* str = reinterpret_cast<const char*>(lParam);
// 将字符串转换为 QString
QString qstr = QString::fromUtf8(str);
// 处理 QString
// ...
return 0;
}
// ...
}
return DefWindowProc(hwnd, msg, wParam, lParam);
}
```
在这个例子中,首先将 `LPARAM` 参数转换为 `const char*` 类型的字符串,然后使用 `QString::fromUtf8` 函数将字符串转换为 `QString` 类型。需要注意的是,这里使用了 `fromUtf8` 函数,因为在 Windows 中,通常使用的是 UTF-8 编码的字符串。
另外,如果你在 `PostMessage` 函数中传递的是 Unicode 字符串,可以使用 `QString::fromWCharArray` 函数将 `LPARAM` 参数中的字符串转换为 `QString`。例如:
```cpp
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_MY_MESSAGE:
{
// 将 LPARAM 参数转换为 Unicode 字符串
const wchar_t* wstr = reinterpret_cast<const wchar_t*>(lParam);
// 将 Unicode 字符串转换为 QString
QString qstr = QString::fromWCharArray(wstr);
// 处理 QString
// ...
return 0;
}
// ...
}
return DefWindowProc(hwnd, msg, wParam, lParam);
}
```
需要注意的是,在使用 `fromWCharArray` 函数时,需要将 `LPARAM` 参数中的字符串转换为 `const wchar_t*` 类型的 Unicode 字符串。
相关推荐
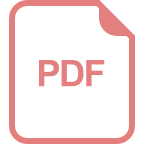
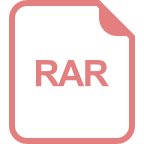
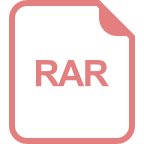













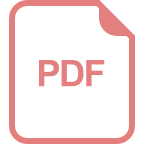
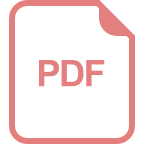