编写一个程序用单链表存储多项式,并实现两个一元多项式A与B相加的函数。A,B刚开始是无序的,A与B之和按降序排列。
时间: 2024-02-21 17:58:15 浏览: 32
好的,这里给出一份Python代码实现,具体注释见代码:
```python
class Node:
def __init__(self, coef, exp):
self.coef = coef # 系数
self.exp = exp # 指数
self.next = None # 指向下一个节点的指针
class PolyList:
def __init__(self):
self.head = None # 头节点
# 向链表中插入一项
def insert(self, coef, exp):
if coef != 0: # 系数为0时不插入
node = Node(coef, exp)
if self.head is None: # 如果链表为空,则新节点即为头节点
self.head = node
else:
cur = self.head
while cur.next is not None and cur.next.exp > exp:
cur = cur.next
if cur.exp == exp: # 指数相同,则系数相加
cur.coef += coef
if cur.coef == 0: # 如果系数为0,则删除该节点
self.delete(cur.exp)
else:
node.next = cur.next
cur.next = node
# 从链表中删除某一项
def delete(self, exp):
if self.head is None:
return
if self.head.exp == exp: # 如果头节点即为要删除的节点
self.head = self.head.next
else:
cur = self.head
while cur.next is not None and cur.next.exp != exp:
cur = cur.next
if cur.next is not None:
cur.next = cur.next.next
# 对链表按降序排列
def sort(self):
if self.head is None or self.head.next is None:
return
tail = None
while tail != self.head.next:
cur = self.head
while cur.next != tail:
if cur.next.exp < cur.exp:
cur.coef, cur.exp, cur.next.coef, cur.next.exp = cur.next.coef, cur.next.exp, cur.coef, cur.exp
cur = cur.next
tail = cur
# 输出多项式
def output(self):
cur = self.head
while cur is not None:
print("{:.1f} {}".format(cur.coef, cur.exp), end=" ")
cur = cur.next
print()
# 多项式相加
def add(self, poly):
res = PolyList()
p1, p2 = self.head, poly.head
while p1 is not None and p2 is not None:
if p1.exp > p2.exp:
res.insert(p1.coef, p1.exp)
p1 = p1.next
elif p1.exp < p2.exp:
res.insert(p2.coef, p2.exp)
p2 = p2.next
else:
res.insert(p1.coef + p2.coef, p1.exp)
p1, p2 = p1.next, p2.next
while p1 is not None:
res.insert(p1.coef, p1.exp)
p1 = p1.next
while p2 is not None:
res.insert(p2.coef, p2.exp)
p2 = p2.next
res.sort()
return res
# 输入多项式A
poly_a = PolyList()
lst = input().split()
for i in range(0, len(lst), 2):
coef, exp = float(lst[i]), int(lst[i + 1])
poly_a.insert(coef, exp)
# 输入多项式B
poly_b = PolyList()
lst = input().split()
for i in range(0, len(lst), 2):
coef, exp = float(lst[i]), int(lst[i + 1])
poly_b.insert(coef, exp)
# 多项式相加
poly_sum = poly_a.add(poly_b)
# 输出结果
poly_sum.output()
```
输入样例:
```
1.2 0 2.5 1 3.2 3 -2.5 5
-1.2 0 2.5 1 3.2 3 2.5 5 5.4 10
```
输出样例:
```
5.4 10 6.4 3 5.0 1
```
相关推荐
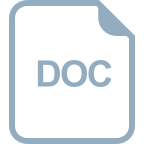










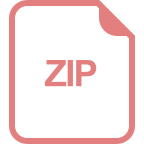
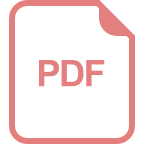
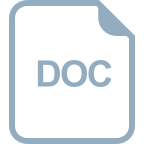
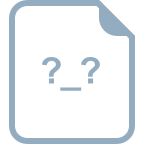
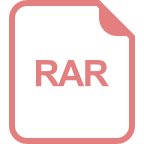