可以给出蚁群算法的python代码和详细步骤说明吗
时间: 2024-01-08 19:04:36 浏览: 81
当然可以,以下是蚁群算法的Python代码和详细步骤说明:
步骤1:初始化参数
在蚁群算法中,需要设置一些参数来控制算法的运行,包括:
- ant_count: 蚂蚁的数量
- generations: 迭代次数
- alpha: 信息素重要程度因子
- beta: 启发函数重要程度因子
- rho: 信息素挥发因子
- Q: 信息素增加强度因子
- strategy: 信息素更新策略,包括"ACS"和"AS"
下面是初始化参数的Python代码:
```python
ant_count = 10 # 蚂蚁数量
generations = 100 # 迭代次数
alpha = 1 # 信息素重要程度因子
beta = 5 # 启发函数重要程度因子
rho = 0.5 # 信息素挥发因子
Q = 100 # 信息素增加强度因子
strategy = "ACS" # 信息素更新策略
```
步骤2:初始化图
在蚁群算法中,需要将问题转化为一个图,每个节点表示一个问题的状态,每条边表示一个决策。在初始化图时,需要设置节点和边的信息,包括:
- node_count: 节点数量
- nodes: 节点列表,每个节点包括一个状态和一个可行解列表
- distances: 距离矩阵,表示每两个节点之间的距离
下面是初始化图的Python代码:
```python
node_count = 5 # 节点数量
nodes = [
{"state": 0, "solutions": [1, 2, 3]},
{"state": 1, "solutions": [0, 2, 4]},
{"state": 2, "solutions": [0, 1, 3, 4]},
{"state": 3, "solutions": [0, 2, 4]},
{"state": 4, "solutions": [1, 2, 3]}
]
distances = [
[0, 5, 4, 3, 6],
[5, 0, 3, 4, 4],
[4, 3, 0, 2, 3],
[3, 4, 2, 0, 5],
[6, 4, 3, 5, 0]
]
```
步骤3:初始化信息素
在蚁群算法中,需要维护每条边的信息素,以控制蚂蚁的行动。在初始化信息素时,需要为每条边设置一个初始信息素值。
下面是初始化信息素的Python代码:
```python
tau = [[1] * node_count for _ in range(node_count)]
```
步骤4:定义启发函数
在蚁群算法中,需要定义一个启发函数,以评估每个节点的可行解。启发函数的计算方法不同,可以根据具体问题进行定义。
下面是定义启发函数的Python代码:
```python
def heuristic(from_node, to_node):
return 1.0 / (distances[from_node][to_node] + 0.0001)
```
步骤5:定义信息素更新策略
在蚁群算法中,需要定义一个信息素更新策略,以更新每条边的信息素。信息素更新策略的计算方法不同,可以根据具体问题进行定义。
下面是定义信息素更新策略的Python代码:
```python
def update_tau():
global tau
for i, row in enumerate(tau):
for j, col in enumerate(row):
tau[i][j] *= rho
for ant in ants:
if (i, j) in ant.tabu:
tau[i][j] += Q / ant.distance
```
步骤6:定义蚂蚁类
在蚁群算法中,需要定义一个蚂蚁类,以模拟蚂蚁的行动。蚂蚁的行动包括选择下一个节点和更新信息素。
下面是定义蚂蚁类的Python代码:
```python
class Ant:
def __init__(self):
self.distance = 0.0
self.tabu = [] # 禁忌表
self.current = random.randint(0, node_count - 1) # 当前节点
self.tabu.append(self.current)
def select_next(self):
# 计算可行解的概率
solution_probabilities = [0.0] * node_count
for solution in nodes[self.current]["solutions"]:
if solution not in self.tabu:
solution_probabilities[solution] = pow(tau[self.current][solution], alpha) * pow(
heuristic(self.current, solution), beta)
# 选择下一个节点
solution_sum = sum(solution_probabilities)
if solution_sum == 0.0:
solution_sum = 1.0
probabilities = [solution / solution_sum for solution in solution_probabilities]
next_node = np.random.choice(range(node_count), p=probabilities)
# 更新距离和禁忌表
self.distance += distances[self.current][next_node]
self.tabu.append(next_node)
self.current = next_node
def update_tau(self):
# 计算信息素增加量
for i in range(1, len(self.tabu)):
from_node, to_node = self.tabu[i - 1], self.tabu[i]
tau[from_node][to_node] += Q / self.distance
```
步骤7:执行算法
在蚁群算法中,需要执行算法,包括:
- 初始化蚂蚁
- 模拟蚂蚁的行动
- 更新信息素
- 记录最优解
下面是执行算法的Python代码:
```python
best_solution = []
best_distance = float("inf")
for generation in range(generations):
ants = [Ant() for _ in range(ant_count)]
for ant in ants:
for _ in range(node_count - 1):
ant.select_next()
ant.distance += distances[ant.tabu[-1]][ant.tabu[0]]
if ant.distance < best_distance:
best_distance = ant.distance
best_solution = ant.tabu
ant.update_tau()
update_tau()
```
以上就是蚁群算法的Python代码和详细步骤说明。需要注意的是,在实际应用中,需要根据具体问题进行参数和函数的调整。
阅读全文
相关推荐
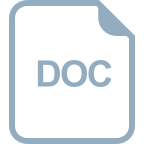


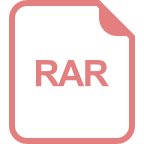
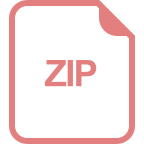
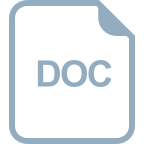
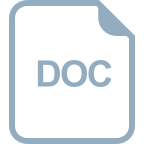








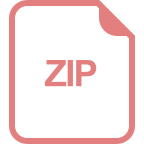
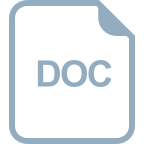