springboot+vue 把本地resource目录下的word转换成html前端展示,word包括文字和图片
时间: 2023-12-06 16:44:56 浏览: 338
首先,你需要使用Java代码将Word文档转换为HTML格式。可以使用Apache POI library来读取和解析Word文档,然后使用Thymeleaf模板引擎来生成HTML代码。
以下是一个简单的示例代码:
```
import java.io.*;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.converter.core.*;
import org.apache.poi.xwpf.converter.xhtml.*;
import org.springframework.core.io.ClassPathResource;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class WordToHtmlController {
@GetMapping("/word-to-html")
public String convertWordToHtml(Model model) throws Exception {
// Load the Word document from the resource folder
File inputFile = new ClassPathResource("word/document.docx").getFile();
InputStream inputStream = new FileInputStream(inputFile);
XWPFDocument document = new XWPFDocument(inputStream);
// Prepare the HTML converter
XHTMLOptions options = XHTMLOptions.create();
OutputStream out = new ByteArrayOutputStream();
XHTMLConverter.getInstance().convert(document, out, options);
// Set the converted HTML content to the model attribute
model.addAttribute("htmlContent", out.toString());
return "word-to-html";
}
}
```
在这个示例中,我们使用了一个简单的Spring Boot控制器来处理`/word-to-html`请求,并将转换后的HTML内容放入模型属性中。我们还使用了`ClassPathResource`来读取位于`src/main/resources/word/document.docx`的Word文档。
接下来,我们需要在前端使用Vue.js来显示转换后的HTML内容。可以使用Vue.js的`v-html`指令来将HTML代码渲染到页面上。以下是一个简单的示例代码:
```
<template>
<div>
<div v-html="htmlContent"></div>
</div>
</template>
<script>
export default {
data() {
return {
htmlContent: ''
}
},
mounted() {
// Load the HTML content from the server
fetch('/word-to-html')
.then(response => response.text())
.then(htmlContent => {
this.htmlContent = htmlContent;
});
}
}
</script>
```
在这个示例中,我们定义了一个Vue.js组件,并使用`v-html`指令将模型属性中的HTML代码渲染到页面上。我们还使用`fetch`函数从服务器加载HTML内容,并将其存储在Vue.js组件的数据属性中。
请注意,这个示例是一个简单的起点。如果你的Word文档包含复杂的格式和布局,你可能需要对代码进行调整以确保正确地转换为HTML格式。
阅读全文
相关推荐
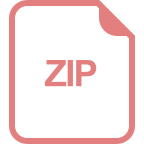
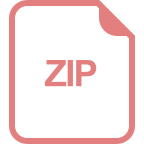
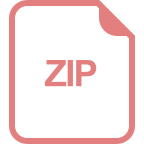
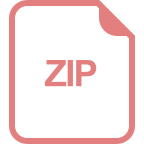
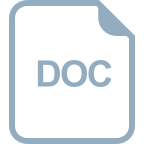
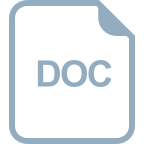
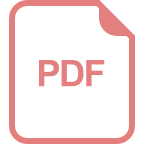
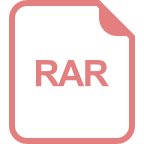
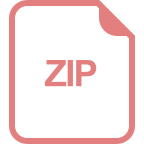
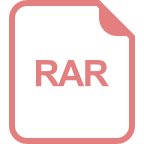
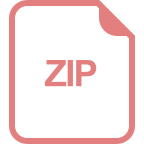
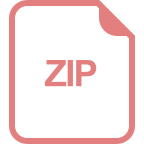
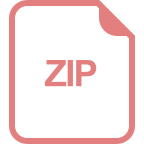
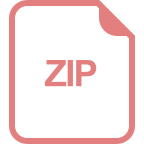
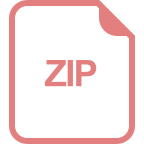
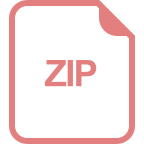