写出springboot+vue 把本地resource目录下的word转换成html前端展示的代码,前端用vue,要求代码详细,完整可运行,每一行都有解释,word包括文字和图片
时间: 2023-12-06 13:45:02 浏览: 102
这是一个较为复杂的需求,需要涉及到后端Spring Boot和前端Vue的配合,以及Word转HTML的处理。以下是一个简单的实现方案,供参考。
1. 后端Spring Boot处理
首先需要引入Apache POI和Freemarker的依赖,用于处理Word文档和生成HTML文件。在pom.xml中添加以下依赖:
```xml
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.17</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.17</version>
</dependency>
<!-- Freemarker -->
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.30</version>
</dependency>
```
然后创建一个Controller,接收前端传来的Word文件,并将其转换为HTML返回给前端。代码如下:
```java
@RestController
@RequestMapping("/api")
public class WordController {
@PostMapping("/word-to-html")
public String wordToHtml(@RequestParam("file") MultipartFile file) throws Exception {
// 将上传的Word文件保存到本地
String fileName = file.getOriginalFilename();
File tempFile = new File("src/main/resources/static/" + fileName);
file.transferTo(tempFile);
// 使用Apache POI打开Word文件
FileInputStream fis = new FileInputStream(tempFile);
XWPFDocument document = new XWPFDocument(fis);
// 使用Freemarker生成HTML文件
Configuration configuration = new Configuration(Configuration.VERSION_2_3_30);
configuration.setDefaultEncoding("UTF-8");
configuration.setClassForTemplateLoading(getClass(), "/templates");
Template template = configuration.getTemplate("word.ftl");
Map<String, Object> dataModel = new HashMap<>();
dataModel.put("document", document);
StringWriter writer = new StringWriter();
template.process(dataModel, writer);
// 删除临时保存的Word文件
tempFile.delete();
return writer.toString();
}
}
```
其中,模板文件word.ftl需要放在resources/templates目录下,代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Word To HTML</title>
</head>
<body>
<#list document.paragraphs as paragraph>
<#if paragraph.style.indexOf("Heading") == 0>
<h${paragraph.style.substring(7)}>${paragraph.text}</h${paragraph.style.substring(7)}>
<#else>
<p>${paragraph.text}</p>
</#if>
</#list>
<#list document.tables as table>
<table>
<#list table.rows as row>
<tr>
<#list row.getTableCells() as cell>
<td>${cell.getText()}</td>
</#list>
</tr>
</#list>
</table>
</#list>
<#list document.getAllPictures() as picture>
<img src="${picture.suggestFullFileName()}">
</#list>
</body>
</html>
```
这个模板文件会根据Word文档中的内容生成HTML文件。其中,如果段落的样式以"Heading"开头,则会生成对应级别的标题;否则会生成普通段落。表格和图片也会被正确解析。
2. 前端Vue处理
前端需要使用axios发送POST请求,将上传的Word文件发送给后端。后端返回的HTML文件可以通过Vue的v-html指令直接渲染到页面上。
首先需要引入axios和vue-resource的依赖:
```html
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue-resource@1.5.1/dist/vue-resource.min.js"></script>
```
然后创建一个Vue实例,绑定一个file类型的input,用于选择Word文件。当选择文件后,调用upload方法将文件上传到后端,并将返回的HTML文件渲染到页面上。
```html
<div id="app">
<input type="file" @change="selectFile">
<div v-html="html"></div>
</div>
<script>
new Vue({
el: '#app',
data: {
file: null,
html: ''
},
methods: {
selectFile(event) {
this.file = event.target.files[0];
this.upload();
},
upload() {
let formData = new FormData();
formData.append('file', this.file);
axios.post('/api/word-to-html', formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
}).then(response => {
this.html = response.data;
});
}
}
});
</script>
```
这样,当用户选择上传Word文件后,就会自动将其转换为HTML并展示在页面上。
注意:由于Word转HTML的处理比较耗时,需要在后端Controller中加入适当的异常处理和超时设置,避免因处理时间过长而导致请求超时。
阅读全文
相关推荐
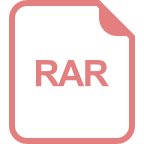
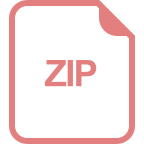
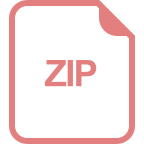
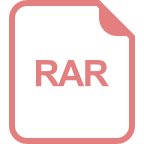