将tensorflow张量中存储的字符串转为python字符串
时间: 2023-11-27 13:09:12 浏览: 81
要将 TensorFlow 张量中存储的字符串转为 Python 字符串,可以使用 TensorFlow 的 `tf.compat.as_str()` 方法。这个方法将字节字符串或 Unicode 字符串转为 Python 字符串。
以下是一个例子:
```python
import tensorflow as tf
# 定义一个 TensorFlow 字符串张量
tensor_str = tf.constant("Hello, TensorFlow!")
# 将 TensorFlow 字符串张量转为 Python 字符串
python_str = tf.compat.as_str(tensor_str.numpy())
print(python_str) # 输出:Hello, TensorFlow!
```
在上面的例子中,我们首先定义了一个 TensorFlow 字符串张量 `tensor_str`,包含 "Hello, TensorFlow!" 这个字符串。然后我们使用 `tf.compat.as_str()` 方法将这个张量转为 Python 字符串,并将结果存入变量 `python_str` 中。最后,我们将这个 Python 字符串打印出来。
相关问题
tensorflow张量如何存储python字符串
在TensorFlow中,可以使用tf.string类型来存储字符串数据,并且可以使用numpy的字符串类型(numpy.string_)将Python字符串转换为TensorFlow字符串。
下面是一个例子:
```python
import tensorflow as tf
import numpy as np
# 创建一个字符串Tensor
string_tensor = tf.constant(["hello", "world", "tensorflow"], dtype=tf.string)
# 将Tensor转换为numpy数组
string_array = string_tensor.numpy()
# 将numpy数组中的字符串类型转换为Python字符串类型
python_string_array = np.char.decode(string_array, 'utf-8')
# 打印Python字符串数组
print(python_string_array)
```
输出:
```
['hello' 'world' 'tensorflow']
```
在这个例子中,我们首先创建一个字符串Tensor,然后将它转换为numpy数组。接着,我们使用numpy.char.decode()函数将字符串类型转换为Python字符串类型,并打印出结果。
tensorflow张量如何添加python字符串
要将Python字符串添加到TensorFlow张量中,您需要将其转换为张量的一部分。可以使用TensorFlow的`tf.constant()`函数将Python字符串转换为常量张量。以下是一个例子:
```
import tensorflow as tf
# Create a string constant tensor
string_tensor = tf.constant("Hello, TensorFlow!")
# Print the string tensor
print(string_tensor)
```
输出:
```
tf.Tensor(b'Hello, TensorFlow!', shape=(), dtype=string)
```
在这个例子中,我们使用`tf.constant()`函数将Python字符串转换为常量张量,并将其存储在变量`string_tensor`中。在打印该变量时,我们可以看到它是一个具有字符串值的张量。请注意,字符串值被编码为字节字符串(`dtype=string`)。
阅读全文
相关推荐
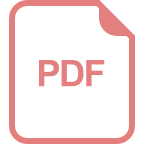
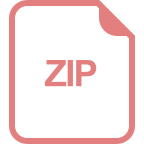
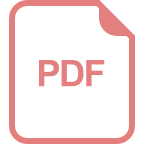

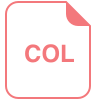
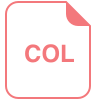

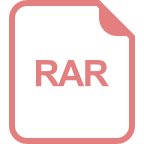
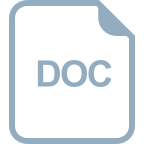
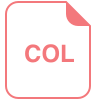
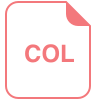





