c++实现分数类fraction利用头文件
时间: 2023-09-24 08:03:35 浏览: 138
以下是使用头文件实现分数类fraction的C++代码示例:
fraction.h头文件:
```c++
#ifndef FRACTION_H
#define FRACTION_H
class Fraction {
public:
Fraction(int n = 0, int d = 1); // 构造函数
Fraction(const Fraction& other); // 复制构造函数
Fraction& operator=(const Fraction& other); // 复制赋值运算符
~Fraction(); // 析构函数
int numerator() const; // 获取分子
int denominator() const; // 获取分母
Fraction operator+(const Fraction& other) const; // 加法运算符
Fraction operator-(const Fraction& other) const; // 减法运算符
Fraction operator*(const Fraction& other) const; // 乘法运算符
Fraction operator/(const Fraction& other) const; // 除法运算符
bool operator==(const Fraction& other) const; // 等于运算符
bool operator!=(const Fraction& other) const; // 不等于运算符
bool operator<(const Fraction& other) const; // 小于运算符
bool operator>(const Fraction& other) const; // 大于运算符
bool operator<=(const Fraction& other) const; // 小于等于运算符
bool operator>=(const Fraction& other) const; // 大于等于运算符
private:
int m_numerator; // 分子
int m_denominator; // 分母
void reduce(); // 约分
};
#endif // FRACTION_H
```
fraction.cpp实现文件:
```c++
#include "fraction.h"
#include <algorithm>
Fraction::Fraction(int n, int d) : m_numerator(n), m_denominator(d) {
reduce();
}
Fraction::Fraction(const Fraction& other) : m_numerator(other.m_numerator), m_denominator(other.m_denominator) {
reduce();
}
Fraction& Fraction::operator=(const Fraction& other) {
if (this != &other) {
m_numerator = other.m_numerator;
m_denominator = other.m_denominator;
reduce();
}
return *this;
}
Fraction::~Fraction() {}
int Fraction::numerator() const {
return m_numerator;
}
int Fraction::denominator() const {
return m_denominator;
}
Fraction Fraction::operator+(const Fraction& other) const {
int n = m_numerator * other.m_denominator + other.m_numerator * m_denominator;
int d = m_denominator * other.m_denominator;
return Fraction(n, d);
}
Fraction Fraction::operator-(const Fraction& other) const {
int n = m_numerator * other.m_denominator - other.m_numerator * m_denominator;
int d = m_denominator * other.m_denominator;
return Fraction(n, d);
}
Fraction Fraction::operator*(const Fraction& other) const {
int n = m_numerator * other.m_numerator;
int d = m_denominator * other.m_denominator;
return Fraction(n, d);
}
Fraction Fraction::operator/(const Fraction& other) const {
int n = m_numerator * other.m_denominator;
int d = m_denominator * other.m_numerator;
return Fraction(n, d);
}
bool Fraction::operator==(const Fraction& other) const {
return m_numerator == other.m_numerator && m_denominator == other.m_denominator;
}
bool Fraction::operator!=(const Fraction& other) const {
return !(*this == other);
}
bool Fraction::operator<(const Fraction& other) const {
return m_numerator * other.m_denominator < other.m_numerator * m_denominator;
}
bool Fraction::operator>(const Fraction& other) const {
return m_numerator * other.m_denominator > other.m_numerator * m_denominator;
}
bool Fraction::operator<=(const Fraction& other) const {
return *this < other || *this == other;
}
bool Fraction::operator>=(const Fraction& other) const {
return *this > other || *this == other;
}
void Fraction::reduce() {
if (m_denominator == 0) {
throw "Denominator cannot be zero.";
}
if (m_denominator < 0) {
m_numerator = -m_numerator;
m_denominator = -m_denominator;
}
int gcd = std::gcd(m_numerator, m_denominator);
m_numerator /= gcd;
m_denominator /= gcd;
}
```
使用示例:
```c++
#include <iostream>
#include "fraction.h"
int main() {
Fraction f1(2, 3);
Fraction f2(1, 4);
Fraction f3 = f1 + f2;
std::cout << f1.numerator() << "/" << f1.denominator() << " + "
<< f2.numerator() << "/" << f2.denominator() << " = "
<< f3.numerator() << "/" << f3.denominator() << std::endl;
return 0;
}
```
阅读全文
相关推荐




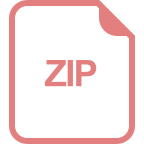













