车牌识别python代码
时间: 2023-07-23 07:12:27 浏览: 48
以下是一个简单的Python车牌识别代码,基于OpenCV和Tesseract OCR库实现:
```
import cv2
import pytesseract
# 读取图片
img = cv2.imread('car.jpg')
# 调整图片大小
img = cv2.resize(img, (620, 480))
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 对图像进行滤波处理
gray = cv2.bilateralFilter(gray, 11, 17, 17)
# 边缘检测
edged = cv2.Canny(gray, 30, 200)
# 查找轮廓
contours, _ = cv2.findContours(edged.copy(), cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 选择最大的轮廓
contours = sorted(contours, key=cv2.contourArea, reverse=True)[:10]
screenCnt = None
# 遍历轮廓
for c in contours:
# 近似轮廓
peri = cv2.arcLength(c, True)
approx = cv2.approxPolyDP(c, 0.018 * peri, True)
# 如果近似轮廓有4个点,就认为是车牌区域
if len(approx) == 4:
screenCnt = approx
break
if screenCnt is None:
detected = 0
print("No contour detected")
else:
detected = 1
if detected == 1:
# 在图像上绘制车牌区域
cv2.drawContours(img, [screenCnt], -1, (0, 255, 0), 3)
# 裁剪车牌区域
mask = np.zeros(gray.shape, np.uint8)
new_image = cv2.drawContours(mask, [screenCnt], 0, 255, -1, )
new_image = cv2.bitwise_and(img, img, mask=mask)
# 转换为灰度图像
new_image_gray = cv2.cvtColor(new_image, cv2.COLOR_BGR2GRAY)
# 图像二值化处理
_, thresh = cv2.threshold(new_image_gray, 110, 255, cv2.THRESH_BINARY)
# 使用Tesseract OCR库识别文字
text = pytesseract.image_to_string(thresh, config='--psm 11')
# 输出识别结果
print("Detected license plate Number is:", text)
# 显示处理后的图像
cv2.imshow('image', img)
cv2.imshow('Cropped Image', thresh)
# 等待按键
cv2.waitKey(0)
```
请注意,这只是一个简单的示例代码。在实际应用中,您可能需要调整一些参数以获得更好的识别结果。
阅读全文
相关推荐
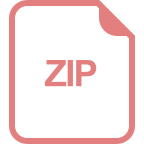












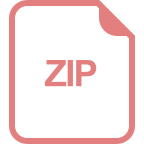
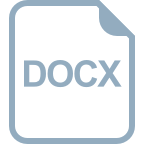