车牌识别python代码示例
时间: 2023-12-05 18:41:22 浏览: 110
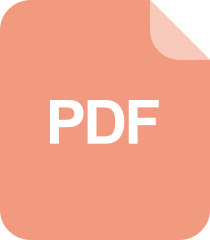
python实现车牌识别的示例代码

以下是两个Python实现车牌识别的示例代码:
1. 基于OpenCV的车牌识别示例代码
```python
import cv2
import numpy as np
# 读取图片
img = cv2.imread('car.jpg')
# 灰度化处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 高斯滤波
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Canny边缘检测
edges = cv2.Canny(blur, 50, 150)
# 轮廓检测
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓
for cnt in contours:
# 计算轮廓面积
area = cv2.contourArea(cnt)
# 过滤面积小于1000的轮廓
if area < 1000:
continue
# 轮廓周长
perimeter = cv2.arcLength(cnt, True)
# 多边形拟合
approx = cv2.approxPolyDP(cnt, 0.02 * perimeter, True)
# 判断是否为矩形
if len(approx) == 4:
# 绘制矩形
cv2.drawContours(img, [approx], 0, (0, 255, 0), 2)
# 显示图片
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2. 基于TensorFlow和CNN的车牌识别示例代码
```python
import tensorflow as tf
import numpy as np
import cv2
# 加载模型
model = tf.keras.models.load_model('model.h5')
# 读取图片
img = cv2.imread('car.jpg')
# 调整图片大小
img = cv2.resize(img, (160, 40))
# 灰度化处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 归一化处理
gray = gray / 255.0
# 转换为三维数组
gray = np.expand_dims(gray, axis=2)
gray = np.expand_dims(gray, axis=0)
# 预测结果
result = model.predict(gray)
# 获取车牌号码
plate_number = ''
for i in range(result.shape[1]):
if result[0][i] < 0.5:
plate_number += '0'
else:
plate_number += '1'
# 显示车牌号码
print('车牌号码:', plate_number)
```
阅读全文
相关推荐
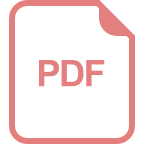













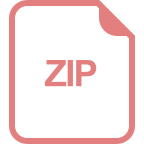