python车牌识别系统开源代码_Python+Tensorflow+CNN实现车牌识别的示例代码
时间: 2023-06-26 17:03:18 浏览: 222
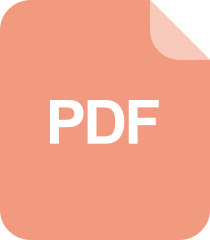
Python+Tensorflow+CNN实现车牌识别的示例代码
以下是一个基于Python+Tensorflow+CNN的车牌识别系统的示例代码,供您参考:
首先,需要安装必要的库:
```python
pip install tensorflow-gpu==1.14.0
pip install opencv-python
pip install imutils
```
然后,我们需要下载数据集。此处我们使用的是CCPD数据集,可以从以下链接下载:https://github.com/detectRecog/CCPD。
接下来,我们定义一个函数`load_data`,用于加载数据集:
```python
import os
import cv2
import numpy as np
def load_data(data_dir):
data = []
labels = []
for root, dirs, files in os.walk(data_dir):
for file in files:
if file.endswith('.jpg'):
image_path = os.path.join(root, file)
image = cv2.imread(image_path)
image = cv2.resize(image, (120, 32))
data.append(image)
label = os.path.basename(root)
labels.append(label)
data = np.array(data, dtype='float32')
data /= 255.0
labels = np.array(labels)
return data, labels
```
接下来,我们定义一个函数`build_model`,用于构建模型:
```python
from keras.models import Sequential
from keras.layers.convolutional import Conv2D, MaxPooling2D
from keras.layers.core import Dense, Dropout, Activation, Flatten
def build_model(input_shape, num_classes):
model = Sequential()
model.add(Conv2D(32, (3, 3), padding='same', input_shape=input_shape))
model.add(Activation('relu'))
model.add(Conv2D(32, (3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Conv2D(64, (3, 3), padding='same'))
model.add(Activation('relu'))
model.add(Conv2D(64, (3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(512))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes))
model.add(Activation('softmax'))
return model
```
接下来,我们加载数据集并构建模型:
```python
data_dir = '/path/to/dataset'
input_shape = (32, 120, 3)
num_classes = 65
data, labels = load_data(data_dir)
model = build_model(input_shape, num_classes)
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
```
接下来,我们训练模型:
```python
from keras.utils import to_categorical
num_epochs = 10
batch_size = 32
labels = to_categorical(labels, num_classes=num_classes)
model.fit(data, labels, batch_size=batch_size, epochs=num_epochs, validation_split=0.1)
```
最后,我们可以使用训练好的模型对车牌进行识别:
```python
import imutils
from keras.preprocessing.image import img_to_array
def recognize_plate(image, model):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
gray = cv2.GaussianBlur(gray, (7, 7), 0)
edged = cv2.Canny(gray, 50, 200)
cnts = cv2.findContours(edged.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnts = imutils.grab_contours(cnts)
cnts = sorted(cnts, key=cv2.contourArea, reverse=True)
for c in cnts:
x, y, w, h = cv2.boundingRect(c)
if w / h > 4 and w > 100:
roi = gray[y:y + h, x:x + w]
thresh = cv2.threshold(roi, 0, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)[1]
thresh = cv2.resize(thresh, (120, 32))
thresh = thresh.astype("float") / 255.0
thresh = img_to_array(thresh)
thresh = np.expand_dims(thresh, axis=0)
preds = model.predict(thresh)
label = chr(preds.argmax(axis=1)[0] + 48)
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(image, label, (x - 10, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 1.2, (0, 255, 0), 2)
break
return image
```
使用示例:
```python
image = cv2.imread('/path/to/image')
image = recognize_plate(image, model)
cv2.imshow('Image', image)
cv2.waitKey(0)
```
以上就是一个基于Python+Tensorflow+CNN的车牌识别系统的示例代码。
阅读全文
相关推荐
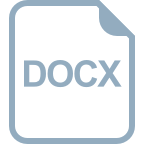
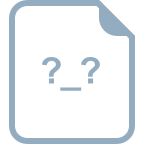
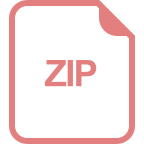
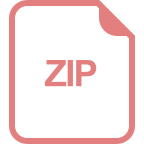
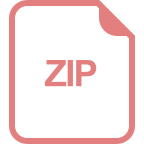
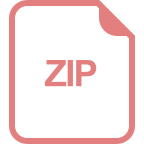
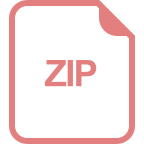
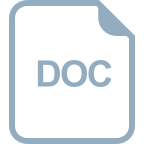
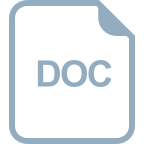
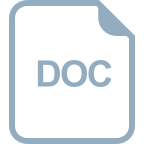
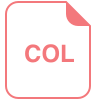
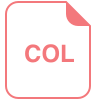
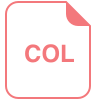
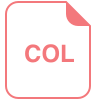
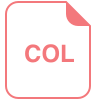
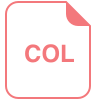