opencv车牌识别python完整全面代码
时间: 2023-07-29 13:08:24 浏览: 89
以下是一份OpenCV车牌识别的Python完整代码。请注意,这只是一个基本示例,您可能需要根据您的具体需求进行更改和优化。
```python
import cv2
import numpy as np
# 车牌识别函数
def plate_recognition(image):
# 图像灰度化
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 高斯滤波
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Sobel算子处理
sobel = cv2.Sobel(blur, cv2.CV_8U, 1, 0, ksize=3)
# 二值化处理
ret, binary = cv2.threshold(sobel, 0, 255, cv2.THRESH_OTSU + cv2.THRESH_BINARY)
# 膨胀和腐蚀操作
element1 = cv2.getStructuringElement(cv2.MORPH_RECT, (30, 9))
element2 = cv2.getStructuringElement(cv2.MORPH_RECT, (24, 6))
dilation = cv2.dilate(binary, element2, iterations=1)
erosion = cv2.erode(dilation, element1, iterations=1)
dilation2 = cv2.dilate(erosion, element2, iterations=3)
# 查找轮廓
contours, hierarchy = cv2.findContours(dilation2, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 筛选车牌轮廓
car_plate = None
for i in range(len(contours)):
cnt = contours[i]
area = cv2.contourArea(cnt)
# 面积筛选
if area < 2000 or area > 50000:
continue
# 轮廓近似
approx = cv2.approxPolyDP(cnt, 4, True)
# 判断是否为矩形
if len(approx) != 4:
continue
# 判断矩形边长比例是否符合要求
x, y, w, h = cv2.boundingRect(cnt)
ratio = float(w) / float(h)
if ratio > 8 or ratio < 2:
continue
# 符合要求的轮廓
car_plate = cnt
break
if car_plate is None:
return None
# 提取车牌图像
x, y, w, h = cv2.boundingRect(car_plate)
car_plate_image = image[y:y+h, x:x+w]
return car_plate_image
# 读取图像
image = cv2.imread('car.jpg')
# 车牌识别
car_plate_image = plate_recognition(image)
# 显示结果
cv2.imshow('Image', image)
cv2.imshow('Car Plate', car_plate_image)
cv2.waitKey(0)
```
在运行此代码之前,请将示例图像保存为“car.jpg”并将其放入与代码相同的目录中。运行代码后,您将看到原始图像和提取的车牌图像。
相关推荐
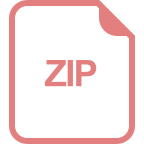
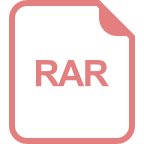
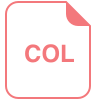












