用C语言和easyx编写聊天机器人的对话界面及其对话框
时间: 2023-06-17 19:05:31 浏览: 231
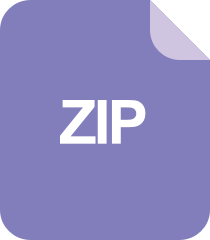
用于创建聊天机器人的快速响应、机器学习、对话式对话引擎,用 Go 编写_代码_下载
以下是使用C语言和EasyX编写的聊天机器人对话框代码:
```c
#include <graphics.h>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 1000
void drawDialogBox(int x, int y, int width, int height, char *title);
void drawText(char *text, int x, int y, int color);
int main()
{
initgraph(640, 480); // 初始化图形界面
char inputText[MAX_LEN]; // 存储用户输入的文本
char outputText[MAX_LEN]; // 存储机器人回复的文本
int x = 50, y = 50; // 对话框位置
int width = 500, height = 300; // 对话框大小
setbkcolor(WHITE); // 设置背景色为白色
cleardevice(); // 清空绘图窗口
// 绘制对话框
drawDialogBox(x, y, width, height, "Chat with Robot");
// 绘制初始提示信息
drawText("Please enter your message:", x + 20, y + 50, BLACK);
while (1)
{
// 获取用户输入
fgets(inputText, MAX_LEN, stdin);
// 清空输入框
clearrectangle(x + 20, y + 70, x + width - 40, y + height - 70);
// 绘制用户输入
drawText(inputText, x + 20, y + 70, BLUE);
// 模拟机器人回复
strcpy(outputText, "Sorry, I am just a demo robot.");
// 清空输出框
clearrectangle(x + 20, y + 120, x + width - 40, y + height - 120);
// 绘制机器人回复
drawText(outputText, x + 20, y + 120, BLACK);
}
getch(); // 等待用户按下任意键
closegraph(); // 关闭图形界面
return 0;
}
// 绘制对话框
void drawDialogBox(int x, int y, int width, int height, char *title)
{
setfillcolor(LIGHTGRAY); // 设置对话框背景色为浅灰色
setlinecolor(BLACK); // 设置对话框边框颜色为黑色
fillrectangle(x, y, x + width, y + height); // 绘制对话框矩形
rectangle(x, y, x + width, y + height); // 绘制对话框边框
drawText(title, x + 10, y + 10, BLACK); // 绘制对话框标题
}
// 绘制文本
void drawText(char *text, int x, int y, int color)
{
settextcolor(color); // 设置文本颜色
outtextxy(x, y, text); // 输出文本
}
```
在上述代码中,我们使用了EasyX提供的绘图函数,包括`initgraph`、`setbkcolor`、`cleardevice`、`setfillcolor`、`setlinecolor`、`fillrectangle`、`rectangle`、`settextcolor`和`outtextxy`等函数,它们可以帮助我们方便地绘制图形界面和文本。
在`main`函数中,我们首先定义了两个字符数组`inputText`和`outputText`,用于存储用户输入的文本和机器人回复的文本。然后,我们定义了对话框的位置、大小和标题,使用`drawDialogBox`函数绘制对话框,并使用`drawText`函数绘制初始提示信息。
在进入循环后,我们使用`fgets`函数获取用户输入的文本,并使用`drawText`函数将其绘制到输入框中。然后,我们模拟机器人回复,使用`drawText`函数将回复文本绘制到输出框中。最后,我们清空输入框和输出框,等待下一轮输入。
需要注意的是,上述代码中使用了`stdin`来获取用户输入的文本,这意味着用户需要在命令行中输入文本,并按下回车键才能使程序进行下一步操作。如果你想要实现更加友好的界面,可以考虑使用EasyX提供的输入框控件,或者调用Windows API中的输入框函数。
阅读全文
相关推荐



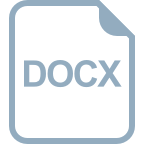
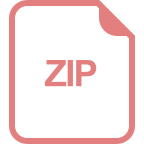
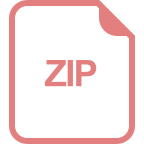








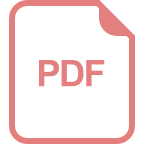