java 地址转GPS
时间: 2023-09-17 07:06:05 浏览: 113
### 回答1:
Java可以使用地理编码服务将地址转换为经纬度坐标(GPS坐标)。地理编码服务是一种将地址转换为经纬度坐标的服务,通常由地图服务提供商提供。
有许多可用于Java的地理编码服务,例如Google Maps API和Bing Maps API。要使用这些服务,您需要注册并获取API密钥。然后,您可以使用Java代码调用API并传递地址信息,API将返回地址的经纬度坐标。
例如,使用Google Maps API进行地址转GPS的示例代码如下所示:
```
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
public class AddressToGPS {
// Replace YOUR_API_KEY with your Google Maps API key
private static final String API_KEY = "YOUR_API_KEY";
public static void main(String[] args) {
String address = "1600 Amphitheatre Parkway, Mountain View, CA";
String latLong = getLatLong(address);
System.out.println(latLong);
}
public static String getLatLong(String address) {
try {
URL url = new URL(
"https://maps.googleapis.com/maps/api/geocode/json?address="
+ address
+ "&key="
+ API_KEY);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Accept", "application/json");
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
String output = "", full = "";
while ((output = br.readLine()) != null) {
full += output;
}
JSONObject jsonObject = new JSONObject(full);
JSONObject jsonObjectLocation =
(JSONObject) jsonObject.get("results")[0].get("geometry").get("location");
### 回答2:
在Java中,地址转GPS坐标可以通过多种方式来实现。以下是一种常见的方法:
1. 使用地理编码服务:Java提供了许多地理编码服务的API,比如Google Maps Geocoding API、百度地图的地理编码API等。可以通过调用这些API来将地址转换为GPS坐标。
示例代码如下:
```java
import org.json.JSONArray;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URL;
import java.net.URLConnection;
import java.net.URLEncoder;
public class AddressToGPSConverter {
public static void main(String[] args) throws Exception {
String address = "北京市朝阳区酒仙桥路10号"; // 待转换的地址
String apiKey = "YOUR_API_KEY"; // 替换为实际的API Key
String encodedAddress = URLEncoder.encode(address, "UTF-8");
String apiUrl = "https://maps.googleapis.com/maps/api/geocode/json?address=" + encodedAddress + "&key=" + apiKey;
URL url = new URL(apiUrl);
URLConnection connection = url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder response = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
JSONObject jsonObject = new JSONObject(response.toString());
JSONArray results = jsonObject.getJSONArray("results");
JSONObject location = results.getJSONObject(0).getJSONObject("geometry").getJSONObject("location");
double latitude = location.getDouble("lat");
double longitude = location.getDouble("lng");
System.out.println("Latitude: " + latitude);
System.out.println("Longitude: " + longitude);
}
}
```
上述代码使用了Google Maps Geocoding API来将地址转换为GPS坐标。需要替换`YOUR_API_KEY`为实际的API Key,以获得访问权限。然后,可以将待转换的地址赋值给`address`变量,并运行代码,即可获得对应的经纬度信息。
另外,还可以使用其他第三方地理编码服务的API,在Java中进行类似的操作。具体使用哪个API取决于需求和个人偏好。
相关推荐
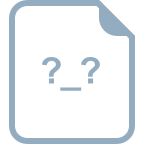














