帮我用c++语言完善下列代码,void BFSTraverse(MGraph G,int v) { //广度优先遍历 }
时间: 2024-02-06 15:11:40 浏览: 126
以下是完善后的代码:
```c++
const int MAX = 100;
bool visited[MAX] = { false };
void BFSTraverse(MGraph G, int v) {
queue<int> Q;
visited[v] = true;
//对顶点v进行操作
Q.push(v);
while (!Q.empty()) {
int i = Q.front();
Q.pop();
for (int j = 0; j < G.vexnum; j++) {
if (G.arcs[i][j] != 0 && !visited[j]) {
visited[j] = true;
//对顶点j进行操作
Q.push(j);
}
}
}
}
```
其中,`BFSTraverse`函数通过队列实现广度优先遍历。首先将起始顶点v标记为已访问,对其进行操作,并将其加入队列。然后对队列中的每个顶点i,遍历其所有未访问的邻接顶点j,并将其标记为已访问,对其进行操作,将其加入队列。直到队列为空,遍历完成。
相关问题
帮我用c++语言完善下列函数,void BFSTraverse(MGraph G,int v) { //广度优先遍历 }
好的,以下是完善后的代码:
```c++
#include <iostream>
#include <queue>
#define MAX_VERTEX_NUM 100
using namespace std;
typedef struct {
// 存放顶点信息的结构体
} VertexType;
typedef struct {
int edges[MAX_VERTEX_NUM][MAX_VERTEX_NUM];
int vexnum, arcnum;
VertexType vexs[MAX_VERTEX_NUM];
} MGraph;
bool visited[MAX_VERTEX_NUM];
void BFSTraverse(MGraph G, int v) {
queue<int> Q;
cout << v << " ";
visited[v] = true;
Q.push(v);
while (!Q.empty()) {
int u = Q.front();
Q.pop();
for (int i = 0; i < G.vexnum; i++) {
if (G.edges[u][i] == 1 && !visited[i]) {
cout << i << " ";
visited[i] = true;
Q.push(i);
}
}
}
}
int main() {
MGraph G;
// 初始化G
BFSTraverse(G, 0);
return 0;
}
```
这段代码实现了广度优先遍历,从顶点v开始遍历。其中,MGraph结构体存储了图的信息,visited数组用于标记顶点是否被访问过。在BFSTraverse函数中,我们用队列来存储待访问的顶点,每次访问一个顶点后,将其未访问的邻居顶点加入队列中,直到队列为空。
帮我用c++语言完善下列程序,#include <iostream> using namespace std; #include "SeqQueue.h" #define INF 9999 //INF无穷大 const int MAX=100; enum GraphType{DG,UG,DN,UN};//图的类型定义:有向图,无向图,有向网,无向网 typedef char VertexType; typedef struct { VertexType vexs[MAX]; //顶点表 int arcs[MAX][MAX]; //邻接矩阵 int vexnum,arcnum; //顶点数和边数 GraphType kind; //图的类型 } MGraph;void BFSTraverse(MGraph G,int v) { //广度优先遍历 }
好的,以下是完善后的代码:
```c++
#include <iostream>
#include "SeqQueue.h"
#define INF 9999 //INF无穷大
const int MAX = 100;
enum GraphType{DG,UG,DN,UN}; //图的类型定义:有向图,无向图,有向网,无向网
typedef char VertexType;
typedef struct {
VertexType vexs[MAX]; //顶点表
int arcs[MAX][MAX]; //邻接矩阵
int vexnum,arcnum; //顶点数和边数
GraphType kind; //图的类型
} MGraph;
bool visited[MAX] = {false}; //标记顶点是否被访问过
void BFSTraverse(MGraph G, int v) { //广度优先遍历
SeqQueue<int> Q(MAX);
cout << v << " ";
visited[v] = true;
Q.EnQueue(v);
while (!Q.IsEmpty()) {
int u;
Q.DeQueue(u);
for (int i = 0; i < G.vexnum; i++) {
if (G.arcs[u][i] != INF && !visited[i]) {
cout << i << " ";
visited[i] = true;
Q.EnQueue(i);
}
}
}
}
int main() {
MGraph G;
// 初始化G
BFSTraverse(G, 0);
return 0;
}
```
这段代码实现了广度优先遍历,从顶点v开始遍历。其中,MGraph结构体存储了图的信息,visited数组用于标记顶点是否被访问过。在BFSTraverse函数中,我们用队列来存储待访问的顶点,每次访问一个顶点后,将其未访问的邻居顶点加入队列中,直到队列为空。注意,在这里我们使用了SeqQueue类,需要引入SeqQueue.h头文件。
阅读全文
相关推荐
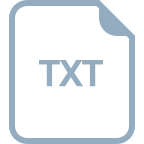
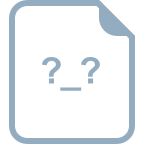
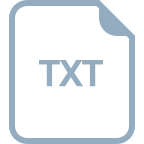







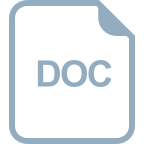
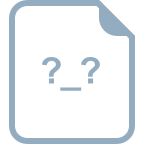
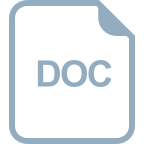
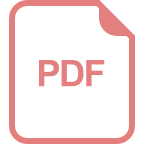
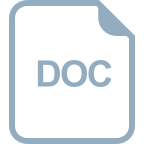
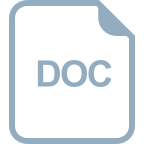