#include<iostream> #include<stack> #include<string> #include<queue> using namespace std; int main() { string str1, str2; stack<char> s; queue<string> print; int i, j; j = 0; cin >> str1 >> str2; for (i = 0; i < str1.size(); i++) { if (str1[i] == str2[j]) { print.push("1->2"); j++; } else if (!s.empty() && s.top() == str2[j]) { s.pop(); print.push("3->2"); j++; i--; } else { s.push(str1[i]); print.push("1->3"); } } while (!s.empty() && s.top() == str2[j] && j < str2.size()) { s.pop(); print.push("3->2"); j++; } if (!s.empty() && s.top() != str2[j]) { cout << "Are you kidding me?" << endl; return 0; } else { while (!print.empty()) { cout << print.front() << endl; print.pop(); } return 0; } } 给这个列车厢调度的代码加上注释
时间: 2024-01-15 16:03:59 浏览: 82
好的,下面是加上注释后的代码:
```c++
#include<iostream>
#include<stack>
#include<string>
#include<queue>
using namespace std;
int main() {
// 定义两个字符串和一个栈和一个队列
string str1, str2;
stack<char> s;
queue<string> print;
int i, j;
j = 0;
// 输入两个字符串
cin >> str1 >> str2;
// 遍历第一个字符串
for (i = 0; i < str1.size(); i++) {
if (str1[i] == str2[j]) { // 如果第一个字符串的当前字符和第二个字符串的当前字符相同
print.push("1->2"); // 将 "1->2" 压入队列
j++; // 移动第二个字符串的指针
}
else if (!s.empty() && s.top() == str2[j]) { // 如果栈不为空,且栈顶元素和第二个字符串的当前字符相同
s.pop(); // 弹出栈顶元素
print.push("3->2"); // 将 "3->2" 压入队列
j++; // 移动第二个字符串的指针
i--; // 回退第一个字符串的指针
}
else { // 如果第一个字符串的当前字符和第二个字符串的当前字符不同
s.push(str1[i]); // 将第一个字符串的当前字符压入栈
print.push("1->3"); // 将 "1->3" 压入队列
}
}
// 处理栈中剩余元素
while (!s.empty() && s.top() == str2[j] && j < str2.size()) {
s.pop(); // 弹出栈顶元素
print.push("3->2"); // 将 "3->2" 压入队列
j++; // 移动第二个字符串的指针
}
// 判断栈是否为空
if (!s.empty() && s.top() != str2[j]) {
cout << "Are you kidding me?" << endl; // 如果栈不为空且栈顶元素和第二个字符串的当前字符不同,输出 "Are you kidding me?"
return 0;
}
else { // 如果栈为空或者栈顶元素和第二个字符串的当前字符相同
while (!print.empty()) { // 输出队列中的操作序列
cout << print.front() << endl;
print.pop();
}
return 0;
}
}
```
希望这份注释能够帮助您理解这段代码的含义。
阅读全文
相关推荐
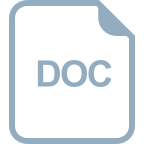
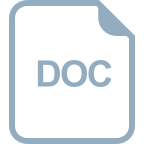
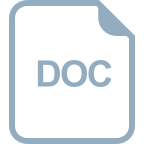











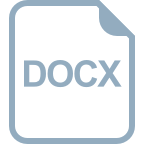
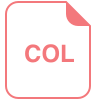
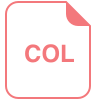
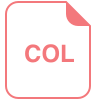
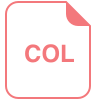