利用栈和队列完成回文的判断代码c++
时间: 2023-12-06 20:05:38 浏览: 105
栈和队列都可以用来实现回文的判断。以下是两种方法的C++代码实现:
使用栈:
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool isPalindrome(string str) {
stack<char> s;
int n = str.length();
int i;
for (i = 0; i < n / 2; i++) {
s.push(str[i]);
}
if (n % 2 != 0) {
i++;
}
while (i < n) {
if (s.top() != str[i]) {
return false;
}
s.pop();
i++;
}
return true;
}
int main() {
string str;
cout << "Enter a string: ";
getline(cin, str);
if (isPalindrome(str)) {
cout << str << " is a palindrome" << endl;
} else {
cout << str << " is not a palindrome" << endl;
}
return 0;
}
```
使用队列:
```cpp
#include <iostream>
#include <queue>
#include <string>
using namespace std;
bool isPalindrome(string str) {
queue<char> q;
int n = str.length();
int i;
for (i = n - 1; i >= n / 2; i--) {
q.push(str[i]);
}
if (n % 2 != 0) {
i--;
}
while (i >= 0) {
if (q.front() != str[i]) {
return false;
}
q.pop();
i--;
}
return true;
}
int main() {
string str;
cout << "Enter a string: ";
getline(cin, str);
if (isPalindrome(str)) {
cout << str << " is a palindrome" << endl;
} else {
cout << str << " is not a palindrome" << endl;
}
return 0;
}
```
阅读全文
相关推荐

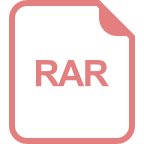

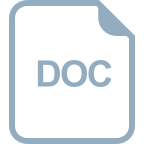











