c 利用栈和队列完成判断一个字符序列是否是回文的函数,并在源程序文件main函数中写测试代码 粘贴回文函数代码: 粘贴源程序main函数测试代码:
时间: 2024-10-24 15:18:44 浏览: 26
为了实现这个功能,我们将创建一个`is_palindrome`函数,该函数接受一个字符序列作为输入,并使用栈来辅助判断是否为回文。以下是`is_palindrome`函数的代码示例:
```c
#include <stdio.h>
#include <stdbool.h>
#include <stack> // 使用C++标准库中的栈
// 定义栈的元素类型
typedef char StackElement;
// 判断字符序列是否为回文的函数
bool is_palindrome(const char* str) {
stack(StackElement) s; // 创建空栈
int len = strlen(str); // 获取字符串长度
// 将字符串的第一个字符逐个压入栈
for (int i = 0; i < len / 2; ++i) {
s.push(str[i]); // 注意:这里只压入一半的字符
}
// 检查另一半是否与栈顶元素一一对应相等
for (int i = len / 2; i < len; ++i) {
if (str[i] != s.top()) {
return false;
}
s.pop(); // 出栈当前元素
}
// 如果到这一步栈为空,说明原序列是回文
return s.empty();
}
// 主函数中的测试代码
int main() {
const char* test_str1 = "madam"; // 测试回文字符串
const char* test_str2 = "hello"; // 测试非回文字符串
printf("Test string 1: %s\n", test_str1);
if (is_palindrome(test_str1)) {
printf("%s is a palindrome.\n", test_str1);
} else {
printf("%s is not a palindrome.\n", test_str1);
}
printf("Test string 2: %s\n", test_str2);
if (is_palindrome(test_str2)) {
printf("%s is a palindrome.\n", test_str2);
} else {
printf("%s is not a palindrome.\n", test_str2);
}
return 0;
}
```
在这个示例中,我们使用栈存储输入字符串的一半字符,然后依次取出并与另一半比较。如果任何时候发现不匹配,则返回false;若遍历完整个字符串且栈为空,说明它是回文。
运行此程序会输出测试字符串1("madam")和2("hello")的判断结果。你可以根据需要添加更多的测试用例。
阅读全文
相关推荐
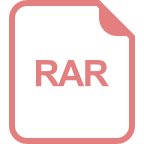
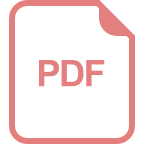
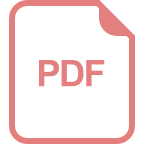















