用C语言用队列编写程序判断 一个字符序列是否是回文。要求:(1)字符序列个数 n可由用户自定义; (2)可连续测试任意多个字符序列,由用户决定退出;(3)字符序列由用户从键盘输入
时间: 2024-02-22 18:59:49 浏览: 63
好的,以下是使用队列实现判断一个字符序列是否为回文的代码及解释。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXLEN 100
typedef struct {
char data[MAXLEN];
int front, rear;
} Queue;
void initQueue(Queue *queue) {
queue->front = queue->rear = 0;
}
int isEmpty(Queue *queue) {
return queue->front == queue->rear;
}
int isFull(Queue *queue) {
return queue->rear == MAXLEN;
}
void enQueue(Queue *queue, char c) {
if (isFull(queue)) {
printf("Queue is full!\n");
exit(EXIT_FAILURE);
}
queue->data[queue->rear++] = c;
}
char deQueue(Queue *queue) {
if (isEmpty(queue)) {
printf("Queue is empty!\n");
exit(EXIT_FAILURE);
}
return queue->data[queue->front++];
}
int isPalindrome(char *str) {
int len = strlen(str);
Queue queue;
initQueue(&queue);
for (int i = 0; i < len; i++) {
enQueue(&queue, str[i]);
}
for (int i = 0; i < len; i++) {
if (deQueue(&queue) != str[len - i - 1]) {
return 0;
}
}
return 1;
}
int main() {
int n;
printf("Input the number of strings: ");
scanf("%d", &n);
char str[MAXLEN];
for (int i = 0; i < n; i++) {
printf("Input string %d: ", i + 1);
scanf("%s", str);
if (isPalindrome(str)) {
printf("String %d is palindrome.\n", i + 1);
} else {
printf("String %d is not palindrome.\n", i + 1);
}
}
return 0;
}
```
这个程序定义了一个队列结构体 `Queue`,包含一个字符数组 `data` 以及队头和队尾的指针 `front` 和 `rear`。程序中实现了队列的基本操作 `initQueue`、`isEmpty`、`isFull`、`enQueue` 和 `deQueue`。
`isPalindrome` 函数用于判断一个字符串是否为回文。它首先计算字符串的长度 `len`,然后将字符串中的字符依次入队,接着再依次出队并和原字符串的对应位置进行比较。如果每个字符都相同,则该字符串为回文,返回值为 1;否则返回值为 0。
在 `main` 函数中,首先从用户输入中读取字符串个数 `n`,然后循环读取每个字符串并调用 `isPalindrome` 函数进行判断。
阅读全文
相关推荐
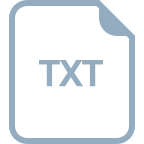















