利用堆栈和队列的性质,通过c语言编写算法函数,实现判断一个字符序列是否是回文,并编写测试主函数进行测试。
时间: 2024-05-02 20:21:52 浏览: 114
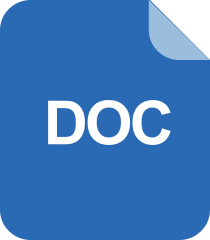
判断字符序列是否是回文

算法函数:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#define MAX_SIZE 100
typedef struct {
char data[MAX_SIZE];
int top;
} Stack;
typedef struct {
char data[MAX_SIZE];
int front;
int rear;
} Queue;
void initStack(Stack *s) {
s->top = -1;
}
bool isStackEmpty(Stack *s) {
return s->top == -1;
}
bool isStackFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, char c) {
if (isStackFull(s)) {
printf("Stack overflow.\n");
return;
}
s->top++;
s->data[s->top] = c;
}
char pop(Stack *s) {
if (isStackEmpty(s)) {
printf("Stack underflow.\n");
return '\0';
}
char c = s->data[s->top];
s->top--;
return c;
}
void initQueue(Queue *q) {
q->front = 0;
q->rear = -1;
}
bool isQueueEmpty(Queue *q) {
return q->front > q->rear;
}
bool isQueueFull(Queue *q) {
return q->rear == MAX_SIZE - 1;
}
void enqueue(Queue *q, char c) {
if (isQueueFull(q)) {
printf("Queue overflow.\n");
return;
}
q->rear++;
q->data[q->rear] = c;
}
char dequeue(Queue *q) {
if (isQueueEmpty(q)) {
printf("Queue underflow.\n");
return '\0';
}
char c = q->data[q->front];
q->front++;
return c;
}
bool isPalindrome(char *str) {
Stack s;
Queue q;
initStack(&s);
initQueue(&q);
int len = strlen(str);
for (int i = 0; i < len; i++) {
char c = str[i];
if (c >= 'A' && c <= 'Z') {
c += 32;
}
if (c >= 'a' && c <= 'z') {
push(&s, c);
enqueue(&q, c);
}
}
while (!isStackEmpty(&s) && !isQueueEmpty(&q)) {
char c1 = pop(&s);
char c2 = dequeue(&q);
if (c1 != c2) {
return false;
}
}
return true;
}
```
测试主函数:
```
int main() {
char str[MAX_SIZE];
printf("Enter a string: ");
scanf("%s", str);
if (isPalindrome(str)) {
printf("%s is a palindrome.\n", str);
} else {
printf("%s is not a palindrome.\n", str);
}
return 0;
}
```
阅读全文
相关推荐
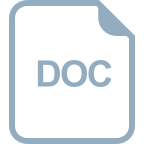
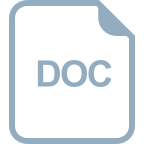















