编写猫类Cat,要求如下:(没有特别要求情况下,均为公共权限) (1)3个属性:品种(type)、昵称(nickname)、年龄(age) ; (2)1个方法:输出Cat所有属性值的方法(printInfo) ; (3)添加如下功能的构造方法无参构造方法,方法体内为各属性赋值, 属性默认值自己确定;带构造方法,让用户实例化对象时只能为“昵称”赋值; 带参构造方法,让用户实例化对象时可以同时为“品种” 、“昵称” 赋值; 带参构造方法,让用户实例化对象时可以同时为“品种”、 “昵称”、 “年龄”赋值; (4)编写测试类TestCat.java测试该类各构造方法的使用。
时间: 2024-03-11 17:49:11 浏览: 16
好的,这是一个简单的猫类Cat的实现:
```java
public class Cat {
// 属性
public String type; // 猫的品种
public String nickname; // 猫的昵称
public int age; // 猫的年龄
// 构造方法
public Cat() {
type = "短毛猫";
nickname = "Tom";
age = 1;
}
public Cat(String nickname) {
this(); // 调用无参构造方法
this.nickname = nickname;
}
public Cat(String type, String nickname) {
this(); // 调用无参构造方法
this.type = type;
this.nickname = nickname;
}
public Cat(String type, String nickname, int age) {
this.type = type;
this.nickname = nickname;
this.age = age;
}
// 方法
public void printInfo() {
System.out.println("品种:" + type);
System.out.println("昵称:" + nickname);
System.out.println("年龄:" + age);
}
}
```
下面是测试类TestCat的实现:
```java
public class TestCat {
public static void main(String[] args) {
// 测试无参构造方法
Cat cat1 = new Cat();
cat1.printInfo();
System.out.println("--------------------");
// 测试带一个参数的构造方法
Cat cat2 = new Cat("Kitty");
cat2.printInfo();
System.out.println("--------------------");
// 测试带两个参数的构造方法
Cat cat3 = new Cat("波斯猫", "Lucy");
cat3.printInfo();
System.out.println("--------------------");
// 测试带三个参数的构造方法
Cat cat4 = new Cat("英国短毛猫", "Lily", 2);
cat4.printInfo();
System.out.println("--------------------");
}
}
```
输出结果如下:
```
品种:短毛猫
昵称:Tom
年龄:1
--------------------
品种:短毛猫
昵称:Kitty
年龄:1
--------------------
品种:波斯猫
昵称:Lucy
年龄:1
--------------------
品种:英国短毛猫
昵称:Lily
年龄:2
--------------------
```
相关推荐
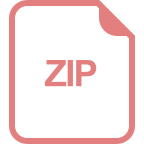
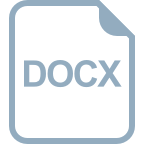
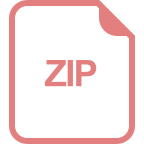















