wpf usercontrol 绑定
时间: 2023-07-31 21:11:29 浏览: 57
WPF UserControl 绑定一般分为两种情况:
1. UserControl 内部控件与 ViewModel 绑定
在 UserControl 内部控件与 ViewModel 绑定时,需要给 UserControl 的 DataContext 属性设置为 ViewModel 的实例,然后在控件内部使用 Binding 绑定控件的属性与 ViewModel 的属性即可。
2. UserControl 的依赖属性绑定
在 UserControl 中定义依赖属性时,可以使用 DependencyProperty.Register 方法来注册依赖属性。然后在使用 UserControl 的地方,就可以使用 Binding 绑定 UserControl 的依赖属性与 ViewModel 的属性。
下面是一个示例:
UserControl XAML 代码:
```xml
<UserControl x:Class="MyUserControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Grid>
<TextBox Text="{Binding Text, Mode=TwoWay}" />
</Grid>
</UserControl>
```
UserControl CodeBehind 代码:
```csharp
public partial class MyUserControl : UserControl
{
public static readonly DependencyProperty TextProperty =
DependencyProperty.Register("Text", typeof(string), typeof(MyUserControl));
public string Text
{
get { return (string)GetValue(TextProperty); }
set { SetValue(TextProperty, value); }
}
public MyUserControl()
{
InitializeComponent();
DataContext = this;
}
}
```
使用 MyUserControl 的地方:
```xml
<Window x:Class="MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:MyNamespace"
Title="MainWindow">
<StackPanel>
<local:MyUserControl Text="{Binding MyText, Mode=TwoWay}" />
<TextBlock Text="{Binding MyText}" />
</StackPanel>
</Window>
```
MainWindow CodeBehind 代码:
```csharp
public partial class MainWindow : Window, INotifyPropertyChanged
{
private string _myText;
public string MyText
{
get { return _myText; }
set
{
_myText = value;
OnPropertyChanged(nameof(MyText));
}
}
public MainWindow()
{
InitializeComponent();
DataContext = this;
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
```
相关推荐
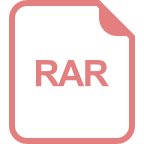
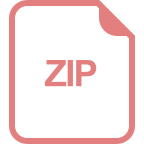














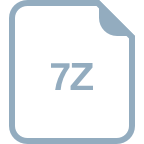