请按照城市的名字(拼音)进行升序排序。 文本文件city.txt中存储了10个城市的名字, (1)请从city.txt中读取这10个城市的名字并输出在屏幕上; (2)然后按照升序排序,把排序结果输出在屏幕上。 c语言
时间: 2024-03-14 12:43:06 浏览: 19
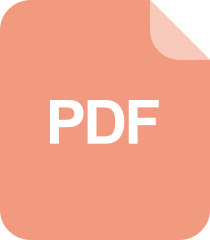
用C语言实现从文本文件中读取数据后进行排序的功能
好的,以下是C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LENGTH 100
int compare(const void *a, const void *b) {
const char **ia = (const char **)a;
const char **ib = (const char **)b;
return strcmp(*ia, *ib);
}
int main() {
char cities[10][MAX_LINE_LENGTH];
int i, j;
FILE *fp = fopen("city.txt", "r");
if (fp == NULL) {
printf("Failed to open file\n");
return 1;
}
// 读取城市列表
for (i = 0; i < 10; i++) {
if (fgets(cities[i], MAX_LINE_LENGTH, fp) == NULL) {
break;
}
cities[i][strcspn(cities[i], "\r\n")] = '\0'; // 去掉换行符
}
// 输出原始城市列表
printf("原始城市列表:\n");
for (i = 0; i < 10; i++) {
printf("%s\n", cities[i]);
}
// 排序并输出
qsort(cities, i, sizeof(cities[0]), compare);
printf("排序后的城市列表:\n");
for (j = 0; j < i; j++) {
printf("%s\n", cities[j]);
}
fclose(fp);
return 0;
}
```
解释一下代码:
首先,我们定义了一个 `compare` 函数,用于比较两个字符串的大小关系。这个函数将被传递给 `qsort` 函数用于排序。
然后,在 `main` 函数中,我们定义了一个二维数组 `cities`,用于存储城市列表。我们使用 `fopen` 函数打开 `city.txt` 文件,并使用 `fgets` 函数读取文件中的每一行城市名称,并将它们存储在 `cities` 数组中。在读取每一行时,我们使用 `strcspn` 函数去掉行末的换行符。
接下来,我们使用一个 `for` 循环遍历 `cities` 数组,并使用 `printf` 函数输出每个城市的名称。
然后,我们使用 `qsort` 函数对城市列表进行排序,这将按照升序对城市进行排序。
最后,我们再次使用 `for` 循环遍历 `cities` 数组,并使用 `printf` 函数输出每个城市的名称。
阅读全文
相关推荐








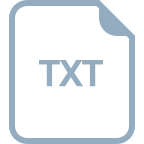
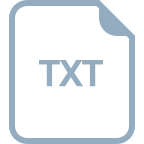


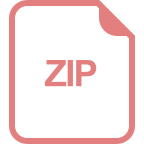