opencv使用dlib库进行人眼检测的c++代码
时间: 2023-09-03 10:25:28 浏览: 77
### 回答1:
下面是使用 OpenCV 和 dlib 库进行人眼检测的 C++ 代码示例:
```c++
#include <dlib/opencv.h>
#include <opencv2/highgui/highgui.hpp>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing/render_face_detections.h>
#include <dlib/image_processing.h>
using namespace dlib;
using namespace std;
int main()
{
// 使用 dlib 的正脸检测器
frontal_face_detector detector = get_frontal_face_detector();
// 使用 dlib 的人脸关键点检测器
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
cv::VideoCapture cap(0);
if (!cap.isOpened())
{
cerr << "Unable to connect to camera" << endl;
return 1;
}
cv::namedWindow("Face Detection");
while (true)
{
cv::Mat temp;
if (!cap.read(temp))
{
break;
}
cv_image<bgr_pixel> cimg(temp);
// 使用正脸检测器检测人脸
std::vector<rectangle> faces = detector(cimg);
// 使用人脸关键点检测器检测关键点
std::vector<full_object_detection> shapes;
for (unsigned long i = 0; i < faces.size(); ++i)
shapes.push_back(sp(cimg, faces[i]));
// 渲染人眼关键点
for (unsigned long i = 0; i < shapes.size(); ++i)
{
cv::circle(temp, cv::Point(shapes[i].part(36).x(), shapes[i].part(36).y()), 2, cv::Scalar(255, 0, 0), -1);
cv::circle(temp, cv::Point(shapes[i].part(45).x(), shapes[i].part(45).y()), 2, cv::Scalar(255, 0, 0), -1);
}
cv::imshow("Face Detection", temp);
if (cv::waitKey(30) == 27)
{
break;
}
}
return 0;
}
```
注意:需要先下载dlib的人脸关键点数据模型并放
### 回答2:
OpenCV是一个开源计算机视觉库,用于处理图像和视频。它提供了许多处理图像和视频的功能。而Dlib是一个C++库,用于进行机器学习和模式识别任务,包括人脸检测和人眼检测。
在使用OpenCV和Dlib进行人眼检测时,需要经过以下几个步骤:
1. 导入所需的库和头文件:
```c++
#include <opencv2/opencv.hpp>
#include <dlib/opencv.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
```
2. 加载图像并转换为灰度图像:
```c++
cv::Mat image = cv::imread("image.jpg");
cv::Mat gray;
cv::cvtColor(image, gray, cv::COLOR_BGR2GRAY);
```
3. 使用Dlib的人脸检测器检测人脸区域:
```c++
dlib::frontal_face_detector detector = dlib::get_frontal_face_detector();
std::vector<dlib::rectangle> faces = detector(dlib::cv_image<unsigned char>(gray));
```
4. 对每个检测到的人脸区域进行人眼检测:
```c++
dlib::shape_predictor sp;
dlib::deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
for (const auto& face : faces) {
dlib::full_object_detection shape = sp(dlib::cv_image<unsigned char>(gray), face);
for (int i = 36; i <= 47; i++) {
cv::circle(image, cv::Point(shape.part(i).x(), shape.part(i).y()), 2, cv::Scalar(0, 255, 0), -1);
}
}
```
5. 显示结果:
```c++
cv::imshow("Eyes Detected", image);
cv::waitKey(0);
```
这段代码首先加载一张图像,并将其转换为灰度图像。然后使用Dlib的人脸检测器检测图像中的人脸区域。接下来,使用Dlib的形状预测器对每个检测到的人脸区域进行人眼检测,并在图像上绘制出人眼的位置。最后,显示处理后的图像。
这就是使用OpenCV和Dlib进行人眼检测的C++代码。当然,还可以根据需要进行一些调整和优化。
### 回答3:
请参考以下示例代码,在使用OpenCV库和dlib库进行人眼检测的C ++代码中,首先需要导入必要的头文件和库文件。
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
#include <dlib/opencv.h>
#include <dlib/image_processing.h>
#include <dlib/image_processing/frontal_face_detector.h>
int main()
{
// 从摄像头捕获图像
cv::VideoCapture cap(0);
// 检查摄像头是否成功打开
if (!cap.isOpened())
{
std::cout << "无法打开摄像头!" << std::endl;
return -1;
}
// 创建窗口来显示图像
cv::namedWindow("人眼检测", cv::WINDOW_NORMAL);
// 初始化dlib的人脸检测器
dlib::frontal_face_detector detector = dlib::get_frontal_face_detector();
// 初始化dlib的眼睛检测器
dlib::shape_predictor predictor;
dlib::deserialize("shape_predictor_68_face_landmarks.dat") >> predictor;
// 持续从摄像头中读取图像
while (true)
{
// 读取图像帧
cv::Mat frame;
cap >> frame;
// 将图像帧转换为dlib图像
dlib::cv_image<dlib::bgr_pixel> dlibImage(frame);
// 用人脸检测器检测人脸位置
std::vector<dlib::rectangle> faces = detector(dlibImage);
// 对每个检测到的人脸进行眼睛检测
for (const auto& face : faces)
{
// 用眼睛检测器检测眼睛位置
dlib::full_object_detection shape = predictor(dlibImage, face);
for (unsigned long i = 36; i <= 41; ++i)
cv::circle(frame, cv::Point(shape.part(i).x(), shape.part(i).y()), 2, cv::Scalar(0, 0, 255), -1);
for (unsigned long i = 42; i <= 47; ++i)
cv::circle(frame, cv::Point(shape.part(i).x(), shape.part(i).y()), 2, cv::Scalar(0, 0, 255), -1);
}
// 显示带有眼睛检测结果的图像帧
cv::imshow("人眼检测", frame);
// 按下ESC键退出程序
if (cv::waitKey(1) == 27)
break;
}
// 释放摄像头和关闭窗口
cap.release();
cv::destroyAllWindows();
return 0;
}
```
这段代码首先创建了一个名为"人眼检测"的窗口,然后从摄像头中读取图像帧,使用dlib人脸检测器检测人脸位置,然后对每个检测到的人脸使用dlib眼睛检测器检测眼睛位置,并在图像上通过绘制红色圆圈来标记眼睛位置。最后,显示包含眼睛检测结果的图像帧,等待按下ESC键来退出程序。
相关推荐
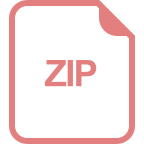
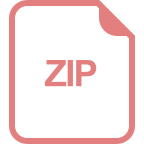
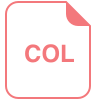
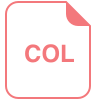
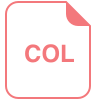
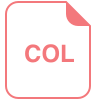
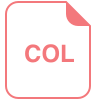








