设计c++语言计算哈夫曼树的WPL值
时间: 2023-10-22 17:15:10 浏览: 149
计算哈夫曼树的WPL(Weighted Path Length)值可以通过以下步骤实现:
1. 定义哈夫曼树节点结构体,包括节点权值、左右子节点指针和父节点指针等成员变量。
```c++
struct HNode {
int weight; // 节点权值
HNode* left; // 左子节点指针
HNode* right; // 右子节点指针
HNode* parent; // 父节点指针
};
```
2. 定义比较函数,用于构建最小堆。
```c++
struct cmp {
bool operator() (const HNode* a, const HNode* b) const {
return a->weight > b->weight;
}
};
```
3. 构建哈夫曼树,首先将每个权值看做一个节点,构建最小堆,然后每次取出堆顶的两个节点,合并成一个新节点,权值为它们的和,直到堆中只剩一个节点,这个节点就是哈夫曼树的根节点。
```c++
int calcWPL(HNode* root) {
if (root == nullptr) return 0;
priority_queue<HNode*, vector<HNode*>, cmp> q;
HNode* p = root;
q.push(p);
while (q.size() > 1) {
HNode* a = q.top();
q.pop();
HNode* b = q.top();
q.pop();
HNode* c = new HNode();
c->weight = a->weight + b->weight;
c->left = a;
c->right = b;
a->parent = c;
b->parent = c;
q.push(c);
}
return calcWPL(root, 0);
}
```
4. 递归计算哈夫曼树的WPL值,从根节点开始,每个节点的WPL值为它到根节点的路径长度乘以它的权值,左右子节点分别递归计算,并将计算结果累加起来。
```c++
int calcWPL(HNode* node, int depth) {
if (node->left == nullptr && node->right == nullptr) {
return depth * node->weight;
}
return calcWPL(node->left, depth + 1) + calcWPL(node->right, depth + 1);
}
```
完整代码如下:
阅读全文
相关推荐
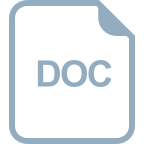
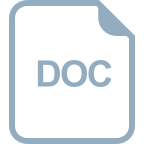
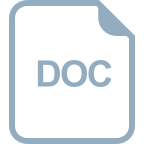








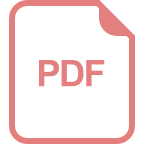
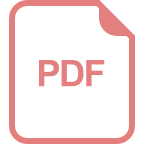
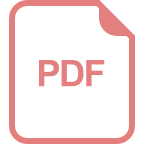
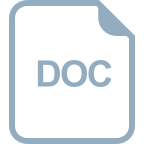



