import seaborn as sns import matplotlib.pyplot as plt from sklearn.metrics import confusion_matrix # Example data y_true = [0, 1, 0, 1, 0, 1, 0, 0, 1, 1] y_pred = [0, 0, 0, 1, 0, 1, 1, 0, 1, 0] # Calculate confusion matrix cm = confusion_matrix(y_true, y_pred) # Plot heatmap sns.heatmap(cm, annot=True, cmap='Blues') plt.xlabel('Predicted labels') plt.ylabel('True labels') plt.show()上述代码中的y_true,y_pred,如何传入自己训练的真实值预测值标签
时间: 2023-09-21 10:08:16 浏览: 53
如果你有自己的训练数据和模型,可以将其预测结果与真实标签分别存储为两个数组,然后将这两个数组传入 `confusion_matrix` 函数中即可得到混淆矩阵。下面是一个示例代码:
```python
import seaborn as sns
import matplotlib.pyplot as plt
from sklearn.metrics import confusion_matrix
# 假设你的真实标签为 y_true,预测标签为 y_pred
y_true = [...] # 真实标签数组
y_pred = [...] # 预测标签数组
# 计算混淆矩阵
cm = confusion_matrix(y_true, y_pred)
# 绘制热力图
sns.heatmap(cm, annot=True, cmap='Blues')
plt.xlabel('Predicted labels')
plt.ylabel('True labels')
plt.show()
```
其中,`y_true` 和 `y_pred` 分别代表真实标签和预测标签的数组,你需要将它们替换为自己的数据即可。注意,这两个数组的长度必须相等。
相关问题
将后面代码绘制的图保存的代码from sklearn.metrics import confusion_matrix import seaborn as sns import matplotlib.pyplot as plt # 预测结果和真实结果 y_true = [0, 1, 2, 0, 1, 2, 0, 1, 2] y_pred = [0, 1, 1, 0, 2, 1, 0, 2, 2] # 计算混淆矩阵 cm = confusion_matrix(y_true, y_pred) # 可视化混淆矩阵 sns.set() sns.heatmap(cm, annot=True, cmap='Blues') plt.xlabel('Predicted label') plt.ylabel('True label') plt.show()
以下是将代码中绘制的图保存到本地的代码:
```python
from sklearn.metrics import confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
# 预测结果和真实结果
y_true = [0, 1, 2, 0, 1, 2, 0, 1, 2]
y_pred = [0, 1, 1, 0, 2, 1, 0, 2, 2]
# 计算混淆矩阵
cm = confusion_matrix(y_true, y_pred)
# 可视化混淆矩阵
sns.set()
sns.heatmap(cm, annot=True, cmap='Blues')
plt.xlabel('Predicted label')
plt.ylabel('True label')
plt.savefig('confusion_matrix.png')
plt.show()
```
这段代码会将绘制的混淆矩阵保存为当前工作路径下的 `confusion_matrix.png` 文件。您可以根据需要修改文件名和路径。
import pandas as pd import numpy as np import scipy.stats as stats import seaborn as sns from sklearn.metrics import RocCurveDisplay from sklearn.model_selection import train_test_split from sklearn.metrics import confusion_matrix,accuracy_score from sklearn.preprocessing import StandardScaler from sklearn.linear_model import LogisticRegression from sklearn import tree from sklearn.decomposition import PCA import matplotlib.pyplot as plt from sklearn.tree import DecisionTreeClassifier请在此基础上续写代码块,要求是(1) 读入数据后,选取自变量"sysBP", "diaBP","age","totChol","BMI", "heartRate", "glucose"记为X,因变量"TenYearCHD"记为y,组成新的数据集。¶
import pandas as pd
import numpy as np
import scipy.stats as stats
import seaborn as sns
from sklearn.metrics import RocCurveDisplay
from sklearn.model_selection import train_test_split
from sklearn.metrics import confusion_matrix,accuracy_score
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
from sklearn import tree
from sklearn.decomposition import PCA
import matplotlib.pyplot as plt
from sklearn.tree import DecisionTreeClassifier
# 读入数据
data = pd.read_csv("heart.csv")
# 选取自变量
X = data[["sysBP", "diaBP", "age", "totChol", "BMI", "heartRate", "glucose"]]
# 因变量
y = data["TenYearCHD"]
# 组成新的数据集
new_data = pd.concat([X, y], axis=1)
# 打印新的数据集
print(new_data.head())
相关推荐
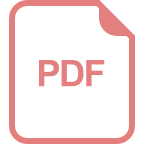
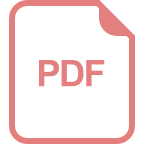
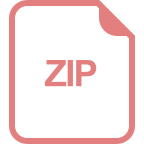












