C语言3.文件“stu_info1.txt”包含学生的基本信息(姓名、学号、籍贯三项),现在需 要从该文件中读出信息,并依次为每位学生输入三门课程成绩,计算平均成绩,再将学生 完整信息(姓名、学号、籍贯、课程 1、课程 2、课程 3、平均分)写入文件 “stu_info2.txt”并保存于磁盘上。
时间: 2023-11-28 17:47:44 浏览: 41
实现这个功能的C语言代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NUM 100 // 学生最大数量
#define MAX_NAME_LEN 20 // 姓名最大长度
#define MAX_NUM_LEN 10 // 学号最大长度
#define MAX_ADDR_LEN 30 // 籍贯最大长度
struct student {
char name[MAX_NAME_LEN]; // 姓名
char num[MAX_NUM_LEN]; // 学号
char addr[MAX_ADDR_LEN]; // 籍贯
float score1, score2, score3; // 三门课程成绩
float avg_score; // 平均成绩
};
int main() {
FILE *fp1, *fp2;
int i, n;
struct student stu[MAX_NUM];
// 打开文件stu_info1.txt读入学生信息
if ((fp1 = fopen("stu_info1.txt", "r")) == NULL) {
printf("File open error!\n");
exit(0);
}
n = 0;
while (fscanf(fp1, "%s%s%s", stu[n].name, stu[n].num, stu[n].addr) != EOF) {
n++;
}
fclose(fp1);
// 依次输入三门课程成绩,计算平均成绩
for (i = 0; i < n; i++) {
printf("Please input the scores of student %s (%s):\n", stu[i].name, stu[i].num);
scanf("%f%f%f", &stu[i].score1, &stu[i].score2, &stu[i].score3);
stu[i].avg_score = (stu[i].score1 + stu[i].score2 + stu[i].score3) / 3;
}
// 将学生完整信息写入文件stu_info2.txt
if ((fp2 = fopen("stu_info2.txt", "w")) == NULL) {
printf("File open error!\n");
exit(0);
}
for (i = 0; i < n; i++) {
fprintf(fp2, "%s %s %s %.2f %.2f %.2f %.2f\n", stu[i].name, stu[i].num, stu[i].addr, stu[i].score1, stu[i].score2, stu[i].score3, stu[i].avg_score);
}
fclose(fp2);
printf("Data has been written into file successfully!\n");
return 0;
}
```
该程序首先读入文件 `stu_info1.txt` 中的学生信息,然后让用户逐一输入每个学生的三门课程成绩,计算平均成绩,并将完整的学生信息(包括姓名、学号、籍贯、三门课程成绩和平均成绩)写入文件 `stu_info2.txt` 中。
相关推荐
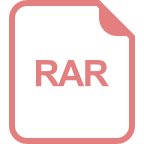













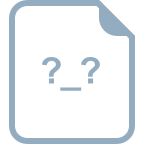