from tkinter import * window = Tk() canvas = Canvas(window, width=500, height=500) canvas.pack() canvas.create_rectangle(50, 50, 100, 150, fill="yellow") canvas.create_rectangle(100, 150, 200, 200, fill="yellow") canvas.create_rectangle(50, 150, 100, 200, fill="yellow") canvas.create_rectangle(50, 200, 100, 300, fill="blue") canvas.create_rectangle(200, 150, 250, 200, fill="yellow") canvas.create_rectangle(200, 200, 250, 300, fill="yellow") canvas.create_rectangle(250, 150, 350, 200, fill="blue") canvas.create_rectangle(50, 300, 100, 350, fill="blue") canvas.create_rectangle(100, 300, 200, 350, fill="blue") canvas.create_rectangle(200, 300, 250, 350, fill="yellow") window.mainloop()这段代码中,如何为这些方块编号
时间: 2023-06-26 13:04:11 浏览: 188
你可以使用 `create_text` 方法在每个方块中心添加编号。例如:
```
canvas.create_text(75, 100, text="1")
canvas.create_text(150, 175, text="2")
canvas.create_text(75, 175, text="3")
canvas.create_text(75, 250, text="4")
canvas.create_text(225, 175, text="5")
canvas.create_text(225, 250, text="6")
canvas.create_text(300, 175, text="7")
canvas.create_text(75, 325, text="8")
canvas.create_text(150, 325, text="9")
canvas.create_text(225, 325, text="10")
```
这将在每个方块中心添加一个文本标签,用于表示它的编号。你可以根据需要将这些坐标调整为更准确的位置。
相关问题
window = tk.Tk() window.title("三维显示系统登录界面") window.geometry("805x660") # 背景画布 import tkinter canvas = tk.Canvas(window,width=10250,height=100) img_gif = tkinter.PhotoImage(file='title3.gif') tkinter.Label(window, image=img_gif).place(relx=-0, rely=0) canvas.pack()sign_in_button = tk.Button(window,text="登录",command=sign_in_f) sign_up_button = tk.Button(window,text="注册",command=sign_up_f) sign_in_button.place(x=350,y=600) sign_up_button.place(x=470,y=600)加入文件调用按钮
下面是修改后的代码,加入了一个按钮,可以让用户选择文件:
```python
import tkinter as tk
from tkinter import filedialog
def sign_in_f():
pass
def sign_up_f():
pass
def open_file():
filename = filedialog.askopenfilename(initialdir="/", title="Select file",
filetypes=(("Text files", "*.txt*"), ("all files", "*.*")))
print(filename)
window = tk.Tk()
window.title("三维显示系统登录界面")
window.geometry("805x660")
# 背景画布
canvas = tk.Canvas(window, width=10250, height=100)
img_gif = tk.PhotoImage(file='title3.gif')
tk.Label(window, image=img_gif).place(relx=-0, rely=0)
canvas.pack()
# 登录和注册按钮
sign_in_button = tk.Button(window, text="登录", command=sign_in_f)
sign_up_button = tk.Button(window, text="注册", command=sign_up_f)
sign_in_button.place(x=350, y=600)
sign_up_button.place(x=470, y=600)
# 文件调用按钮
file_button = tk.Button(window, text="选择文件", command=open_file)
file_button.place(x=600, y=600)
window.mainloop()
```
在这个例子中,我们定义了一个新的函数`open_file()`,当用户点击文件按钮时,调用该函数打开文件对话框。当用户选择文件后,文件的路径会打印到控制台上。你可以根据需要修改`open_file()`函数的实现,以便在GUI中显示所选文件的路径。
from math import * from tkinter import * root=Tk() w=Canvas(root,width=600,height=600) w.pack() w0=300 h0=300 w.create_line(0,300,600,300,fill="red") w.create_line(300,0,300,600,fill="red") def x(t): x=80*(2*sin(t)-sin(2*t)) x+=w0 return x def y(t): y=80*(2*cos(t)-cos(2*t)) return y t=-pi while t<pi: w.create_line(x(t),y(t),x(t+0.01),y(t+0.01),fill="blue") t+=0.01 root.mainloop()上述代码,画出来倒置的爱心,是哪里出了问题
在上述代码中,出现了倒置的爱心,这是因为在计算 y 坐标时,应该使用 sin 函数,而不是 cos 函数。具体来说,在函数 y(t) 中,应该是:
```python
def y(t):
y = 80 * (2 * sin(t) - sin(2 * t))
y += h0
return y
```
而不是:
```python
def y(t):
y = 80 * (2 * cos(t) - cos(2 * t))
return y
```
这样修改之后,代码应该是这样的:
```python
from math import *
from tkinter import *
root = Tk()
w = Canvas(root, width=600, height=600)
w.pack()
w0 = 300
h0 = 300
w.create_line(0, 300, 600, 300, fill="red")
w.create_line(300, 0, 300, 600, fill="red")
def x(t):
x = 80 * (2 * sin(t) - sin(2 * t))
x += w0
return x
def y(t):
y = 80 * (2 * sin(t) - sin(2 * t))
y += h0
return y
t = -pi
while t < pi:
w.create_line(x(t), y(t), x(t + 0.01), y(t + 0.01), fill="blue")
t += 0.01
root.mainloop()
```
这样就可以正确绘制出正向的爱心曲线了。
阅读全文
相关推荐
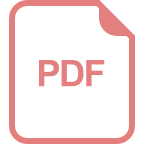
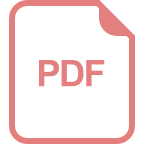
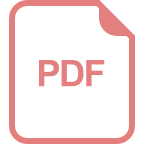













