pytorch使用biLSTM实现一维语音分类代码
时间: 2023-12-09 08:04:26 浏览: 46
以下是一个简单的基于pytorch使用biLSTM实现一维语音分类的代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
# 构建BiLSTM模型
class BiLSTM(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, num_classes):
super(BiLSTM, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True, bidirectional=True)
self.fc = nn.Linear(hidden_size*2, num_classes)
def forward(self, x):
h0 = torch.zeros(self.num_layers*2, x.size(0), self.hidden_size).to(device)
c0 = torch.zeros(self.num_layers*2, x.size(0), self.hidden_size).to(device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
# 超参数设置
input_size = 1
hidden_size = 64
num_layers = 2
num_classes = 10
batch_size = 64
learning_rate = 0.001
num_epochs = 10
# 准备数据
train_data = np.load('train_data.npy')
train_labels = np.load('train_labels.npy')
train_data = np.expand_dims(train_data, axis=2)
train_labels = torch.from_numpy(train_labels).long()
train_dataset = torch.utils.data.TensorDataset(torch.from_numpy(train_data), train_labels)
train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
# 定义设备
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# 初始化模型、损失函数和优化器
model = BiLSTM(input_size, hidden_size, num_layers, num_classes).to(device)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
# 训练模型
for epoch in range(num_epochs):
for i, (data, labels) in enumerate(train_loader):
data = data.to(device)
labels = labels.to(device)
outputs = model(data)
loss = criterion(outputs, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (i+1) % 10 == 0:
print('Epoch [{}/{}], Step [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, i+1, len(train_loader), loss.item()))
# 测试模型
model.eval()
test_data = np.load('test_data.npy')
test_labels = np.load('test_labels.npy')
test_data = np.expand_dims(test_data, axis=2)
test_labels = torch.from_numpy(test_labels).long()
test_dataset = torch.utils.data.TensorDataset(torch.from_numpy(test_data), test_labels)
test_loader = torch.utils.data.DataLoader(test_dataset, batch_size=batch_size, shuffle=False)
with torch.no_grad():
correct = 0
total = 0
for data, labels in test_loader:
data = data.to(device)
labels = labels.to(device)
outputs = model(data)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Test Accuracy of the model on the {} test images: {} %'.format(total, 100 * correct / total))
```
在这个例子中,我们使用了一个由两个双向LSTM层组成的模型,并将其应用于一维语音分类任务。我们使用Adam优化器来更新模型参数,并使用交叉熵损失函数来计算损失。在训练完成后,我们在测试集上评估模型的准确率。
相关推荐
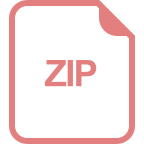
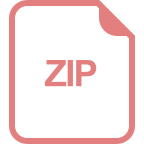














