#include <stdio.h> #include <stdlib.h> #include <string.h> #include <openssl/des.h> #define BLOCK_SIZE 8 void increment_iv(unsigned char *iv) { int i; for (i = BLOCK_SIZE - 1; i >= 0; i--) { if (iv[i] == 0xff) { iv[i] = 0; } else { iv[i]++; break; } }} void des_ctr_encrypt(unsigned char *plaintext, unsigned char *ciphertext, long plaintext_len, unsigned char *key, unsigned char *iv) { DES_key_schedule key_schedule; DES_set_key_checked(key, &key_schedule); unsigned char keystream[BLOCK_SIZE]; unsigned char block[BLOCK_SIZE]; int i, j; for (i = 0; i < plaintext_len; i += BLOCK_SIZE) { DES_ecb_encrypt(iv, keystream, &key_schedule, DES_ENCRYPT); for (j = 0; j < BLOCK_SIZE; j++) { block[j] = plaintext[i + j] ^ keystream[j]; ciphertext[i + j] = block[j]; } increment_iv(iv); } } void des_ctr_decrypt(unsigned char *ciphertext, unsigned char *plaintext, long ciphertext_len, unsigned char *key, unsigned char *iv) { DES_key_schedule key_schedule; DES_set_key_checked(key, &key_schedule); unsigned char keystream[BLOCK_SIZE]; unsigned char block[BLOCK_SIZE]; int i, j; for (i = 0; i < ciphertext_len; i += BLOCK_SIZE) { DES_ecb_encrypt(iv, keystream, &key_schedule, DES_ENCRYPT); for (j = 0; j < BLOCK_SIZE; j++) { block[j] = ciphertext[i + j] ^ keystream[j]; plaintext[i + j] = block[j]; } increment_iv(iv); }} int main() { unsigned char key[] = "01234567"; unsigned char iv[] = "12345678"; unsigned char plaintext[] = "Hello, DES-CTR!"; long plaintext_len = strlen((char *) plaintext); unsigned char ciphertext[plaintext_len]; des_ctr_encrypt(plaintext, ciphertext, plaintext_len, key, iv); printf("Ciphertext: %s\n", ciphertext); unsigned char decrypted[plaintext_len]; des_ctr_decrypt(ciphertext, decrypted, plaintext_len, key, iv); printf("Plaintext: %s\n", decrypted); return 0;}
时间: 2024-04-21 15:26:37 浏览: 131
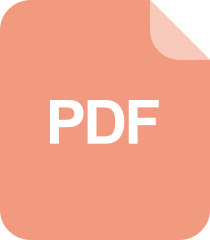
C标准库函数集

这段代码实现了基于 DES 算法的 CTR 模式加密和解密功能。CTR 模式是一种流密码模式,在加密过程中,明文按照固定的块长(这里是 8 字节)被分成若干块,每个块都会被加密。CTR 模式需要使用一个初始向量 IV 和密钥 key,通过 DES 算法将 IV 加密得到一个密钥流 keystream,然后将明文按块与 keystream 进行异或操作得到密文,同时 IV 也需要进行增量操作,保证下一次加密时使用的 IV 是不同的。解密过程与加密过程类似,只需要将密文按块与 keystream 进行异或操作得到明文即可。在这个例子中,输入的明文是 "Hello, DES-CTR!",输出的密文和解密后的明文会在命令行中显示。
阅读全文
相关推荐
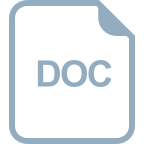
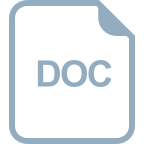














