np.uint8(np.clip((retinex+3)*64, 0, 255))
时间: 2023-11-16 21:05:15 浏览: 34
这行代码中,np.clip函数用来将数组中的值限制在一个范围内。这里将(retinex+3)*64的结果限制在0到255之间,然后将结果转换为无符号8位整型(np.uint8)。这个操作的目的是将(retinex+3)*64的结果缩放到0到255之间,并将其转换为整数类型,以便于进行后续的处理和显示。
相关问题
怎么解决C:\Users\hp\venv\Scripts\python.exe "D:\BI she\pythonProject3\MSRCR.py" Traceback (most recent call last): File "D:\BI she\pythonProject3\MSRCR.py", line 31, in <module> img_retinex = retinex_with_color_restoration(img) File "D:\BI she\pythonProject3\MSRCR.py", line 20, in retinex_with_color_restoration img_retinex = np.uint8(np.clip((img_retinex * img_msb) / 255.0, 0, 255)) ValueError: operands could not be broadcast together with shapes (1080,1440,3) (1080,1440)
这个报错是因为 `img_retinex` 和 `img_msb` 的形状不匹配导致的。根据代码,可以发现 `img_msb` 是一个二维数组,而 `img_retinex` 是一个三维数组。在计算 `(img_retinex * img_msb) / 255.0` 时,两个数组的形状不匹配,因此会报错。
为了解决这个问题,可以将 `img_msb` 扩展为与 `img_retinex` 相同的形状,即在第三个维度上复制两次。具体方法如下:
```python
img_msb = np.expand_dims(img_msb, axis=2)
img_msb = np.tile(img_msb, [1, 1, 3])
```
将上述代码添加到 `retinex_with_color_restoration()` 函数中,在计算 `(img_retinex * img_msb) / 255.0` 之前,即可解决这个问题。
完整代码示例:
```python
import cv2
import numpy as np
def retinex_with_color_restoration(img, sigma_list=[15, 80, 250], G=5, b=25, alpha=125, beta=46, low_clip=0.01, high_clip=0.99):
img = np.float64(img) + 1.0
img_retinex = np.zeros_like(img)
for sigma in sigma_list:
img_retinex += np.log10(img) - np.log10(cv2.GaussianBlur(img, (G, G), sigma))
img_color = np.zeros_like(img)
for channel in range(3):
img_color[:, :, channel] = np.median(img[:, :, channel])
img_msb = np.max(img_color, axis=2)
img_msb = (cv2.GaussianBlur(img_msb, (b, b), sigma_list[0]) + alpha) * 255 / (beta + alpha)
img_msb = np.expand_dims(img_msb, axis=2)
img_msb = np.tile(img_msb, [1, 1, 3])
img_retinex = cv2.normalize(img_retinex, None, 0, 255, cv2.NORM_MINMAX)
img_retinex = np.uint8(np.clip((img_retinex * img_msb) / 255.0, 0, 255))
low_val, high_val = np.percentile(img_retinex, [low_clip * 100, high_clip * 100])
img_retinex = np.uint8(cv2.normalize(img_retinex, None, low_val, high_val, cv2.NORM_MINMAX))
return img_retinex
# 读取图像
img = cv2.imread('example.jpg')
# 进行带色彩恢复因子的Retinex算法亮度增强
img_retinex = retinex_with_color_restoration(img)
# 显示结果
cv2.imshow('Original', img)
cv2.imshow('Retinex', img_retinex)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码示例中,`np.expand_dims()` 函数用于在第二个维度上添加一个长度为1的维度,将 `img_msb` 转换为二维数组,然后使用 `np.tile()` 函数将其复制两次,以匹配 `img_retinex` 的形状。
参数应该写多少def MSRCR(img, sigma_list, G, b, alpha, beta, low_clip, high_clip): img = np.float64(img) + 1.0 img_retinex = multiScaleRetinex(img, sigma_list) img_color = colorRestoration(img, alpha, beta) img_msrcr = G * (img_retinex * img_color + b) for i in range(img_msrcr.shape[2]): img_msrcr[:, :, i] = (img_msrcr[:, :, i] - np.min(img_msrcr[:, :, i])) / \ (np.max(img_msrcr[:, :, i]) - np.min(img_msrcr[:, :, i])) * \ 255 img_msrcr = np.uint8(np.minimum(np.maximum(img_msrcr, 0), 255)) img_msrcr = simplestColorBalance(img_msrcr, low_clip, high_clip) return img_msrcr
这个函数需要的参数包括:
- img: 需要进行 MSRCR 的图像,类型为 numpy 数组。
- sigma_list: multiScaleRetinex 函数中使用的高斯核的标准差列表,类型为列表。
- G: 调整图像对比度的参数,类型为 float。
- b: 调整图像亮度的参数,类型为 float。
- alpha: colorRestoration 函数中使用的参数,类型为 float。
- beta: colorRestoration 函数中使用的参数,类型为 float。
- low_clip: simplestColorBalance 函数中使用的参数,控制最暗像素的值,类型为 float。
- high_clip: simplestColorBalance 函数中使用的参数,控制最亮像素的值,类型为 float。
因此,定义的函数应该为:
```python
def MSRCR(img, sigma_list, G, b, alpha, beta, low_clip, high_clip):
img = np.float64(img) + 1.0
img_retinex = multiScaleRetinex(img, sigma_list)
img_color = colorRestoration(img, alpha, beta)
img_msrcr = G * (img_retinex * img_color + b)
for i in range(img_msrcr.shape[2]):
img_msrcr[:, :, i] = (img_msrcr[:, :, i] - np.min(img_msrcr[:, :, i])) / \
(np.max(img_msrcr[:, :, i]) - np.min(img_msrcr[:, :, i])) * \
255
img_msrcr = np.uint8(np.minimum(np.maximum(img_msrcr, 0), 255))
img_msrcr = simplestColorBalance(img_msrcr, low_clip, high_clip)
return img_msrcr
```
相关推荐
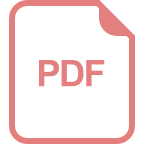












