//总总量 BigDecimal deliveryWgt = new BigDecimal(0); List<StkPrdMaterialInfoVO> prdInfoOfWgt = stkprdPrdInfoService.selectWgtByRlotnoAndPrdNo( details, deliveryInfoDTOShip.getDeliveryCode()); List<StkPrdMaterialInfoVO> tldMXOfWgt = stkprdLcWlTldMxService.getWlTldMXWaitWgt( model.getPickupCode()); //校验数据 Map<String, StkPrdMaterialInfoVO> prdInfoMap = new HashMap<>(2); //根据物料编码把配货单重量转换成map结构 for (StkPrdMaterialInfoVO mater : prdInfoOfWgt) { prdInfoMap.put(mater.getMatCode(), mater); deliveryWgt = deliveryWgt.add(mater.getActWgt()); } //根据物料编码把提料单明细重量转换成map结构 Map<String, StkPrdMaterialInfoVO> tldMXMap = new HashMap<>(2); for (StkPrdMaterialInfoVO mater : tldMXOfWgt) { tldMXMap.put(mater.getMatCode(), mater); } //物料相同的重量进行比对 for (Map.Entry<String, StkPrdMaterialInfoVO> entry : prdInfoMap.entrySet()) { String key = entry.getKey(); StkPrdMaterialInfoVO mater = entry.getValue(); StkPrdMaterialInfoVO mater1 = tldMXMap.get(key); if (mater.getActWgt().compareTo(mater1.getWaitWgt()) > 0) { throw new CustomException(model.getPickupCode() + "提料单超出待发运重量,保存失败!"); } }优化
时间: 2024-02-14 16:36:13 浏览: 10
这段代码可以进行以下优化:
1.在循环前,可以将 prdInfoOfWgt 和 tldMXOfWgt 中的物料按照物料编码排序,这样可以减少后面的查找次数。
2.可以使用 BigDecimal 的 compareTo 方法来进行比较,而不是使用 if 判断。
3.可以将两个 map 的创建提到循环前面,避免在循环中重复创建。
4.可以使用 try-catch 块来捕获异常,而不是抛出自定义异常。
相关问题
//总总量 BigDecimal deliveryWgt = new BigDecimal(0); List<StkPrdMaterialInfoVO> prdInfoOfWgt = stkprdPrdInfoService.selectWgtByRlotnoAndPrdNo( details, deliveryInfoDTOShip.getDeliveryCode()); List<StkPrdMaterialInfoVO> tldMXOfWgt = stkprdLcWlTldMxService.getWlTldMXWaitWgt( model.getPickupCode()); //校验数据 Map<String, StkPrdMaterialInfoVO> prdInfoMap = new HashMap<>(2); //根据物料编码把配货单重量转换成map结构 for (StkPrdMaterialInfoVO mater : prdInfoOfWgt) { prdInfoMap.put(mater.getMatCode(), mater); deliveryWgt = deliveryWgt.add(mater.getActWgt()); } //根据物料编码把提料单明细重量转换成map结构 Map<String, StkPrdMaterialInfoVO> tldMXMap = new HashMap<>(2); for (StkPrdMaterialInfoVO mater : tldMXOfWgt) { tldMXMap.put(mater.getMatCode(), mater); } //物料相同的重量进行比对 for (Map.Entry<String, StkPrdMaterialInfoVO> entry : prdInfoMap.entrySet()) { String key = entry.getKey(); StkPrdMaterialInfoVO mater = entry.getValue(); StkPrdMaterialInfoVO mater1 = tldMXMap.get(key); if (mater.getActWgt().compareTo(mater1.getWaitWgt()) > 0) { throw new CustomException(model.getPickupCode() + "提料单超出待发运重量,保存失败!"); } }优化后的完整代码
//总总量
BigDecimal deliveryWgt = new BigDecimal(0);
//获取配货单重量信息
List<StkPrdMaterialInfoVO> prdInfoOfWgt = stkprdPrdInfoService.selectWgtByRlotnoAndPrdNo(details, deliveryInfoDTOShip.getDeliveryCode());
//获取提料单重量信息
List<StkPrdMaterialInfoVO> tldMXOfWgt = stkprdLcWlTldMxService.getWlTldMXWaitWgt(model.getPickupCode());
//将配货单重量信息和提料单重量信息转换成 map 结构
Map<String, StkPrdMaterialInfoVO> prdInfoMap = new HashMap<>(2);
Map<String, StkPrdMaterialInfoVO> tldMXMap = new HashMap<>(2);
for (StkPrdMaterialInfoVO mater : prdInfoOfWgt) {
prdInfoMap.put(mater.getMatCode(), mater);
deliveryWgt = deliveryWgt.add(mater.getActWgt());
}
for (StkPrdMaterialInfoVO mater : tldMXOfWgt) {
tldMXMap.put(mater.getMatCode(), mater);
}
//物料相同的重量进行比对
for (Map.Entry<String, StkPrdMaterialInfoVO> entry : prdInfoMap.entrySet()) {
String key = entry.getKey();
StkPrdMaterialInfoVO mater = entry.getValue();
StkPrdMaterialInfoVO mater1 = tldMXMap.get(key);
try {
mater.getActWgt().compareTo(mater1.getWaitWgt());
} catch (NullPointerException e) {
throw new CustomException(model.getPickupCode() + "提料单数据错误,保存失败!");
}
if (mater.getActWgt().compareTo(mater1.getWaitWgt()) > 0) {
throw new CustomException(model.getPickupCode() + "提料单超出待发运重量,保存失败!");
}
}
List<BigDecimal> priceList = new ArrayList<>(); 获取平均值
可以使用 BigDecimal 进行精确计算,具体方法如下:
1. 定义 BigDecimal 类型的 sum 变量,并初始化为 0。
2. 遍历 priceList 中的每个元素,将其加入到 sum 变量中。
3. 使用 BigDecimal 类的 divide 方法,将 sum 变量除以 priceList 的大小(即元素个数),得到平均值。
示例代码如下:
```
List<BigDecimal> priceList = new ArrayList<>();
// 将元素添加到 priceList 中
BigDecimal sum = new BigDecimal(0);
for (BigDecimal price : priceList) {
sum = sum.add(price); // 将元素加入到 sum 中
}
BigDecimal average = sum.divide(new BigDecimal(priceList.size()), 2, RoundingMode.HALF_UP);
// 将 sum 除以列表大小,得到平均值,小数位数为 2,舍入方式为四舍五入
System.out.println("平均值为:" + average);
```
相关推荐
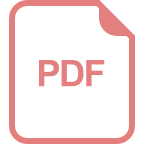
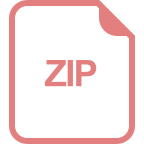













