解释如下代码: @PostMapping("/setManager/{id}/{admin}") @ResponseBody public void setManager(@PathVariable("id")long id, @PathVariable("admin")String admin){ User user = new User(); user.setId(id); user.setAdmin(admin); userService.setManager(user); }
时间: 2023-06-11 16:04:52 浏览: 91
这段代码是一个用于设置用户管理员的接口。通过使用 @PostMapping 注解来标识 HTTP POST 请求,使用 @PathVariable 注解来获取请求 URL 中的参数 id 和 admin,分别表示用户的唯一标识和管理员身份。接着,通过实例化一个 User 对象并设置其 id 和 admin 属性,最后调用 userService 类的 setManager 方法来将用户的管理员属性设置为 admin。该接口没有返回结果,使用 @ResponseBody 注解表明返回的数据被写入 HTTP 响应正文。
相关问题
在springboot用@Controller @ResponseBody@Autowired @PostMapping@Service@Mapper@Data实现用户登录案例
好的,下面是一个基于 Spring Boot 的用户登录案例:
1. 创建 User 实体类
```java
@Data
public class User {
private String username;
private String password;
}
```
2. 创建 UserService 接口和实现类
```java
public interface UserService {
User login(String username, String password);
}
@Service
public class UserServiceImpl implements UserService {
@Override
public User login(String username, String password) {
// 在这里校验用户名和密码是否正确,省略实现
return new User(username, password);
}
}
```
3. 创建 UserController 控制器
```java
@Controller
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/login")
@ResponseBody
public String login(@RequestBody User user) {
User loginUser = userService.login(user.getUsername(), user.getPassword());
if (loginUser != null) {
return "登录成功";
} else {
return "用户名或密码错误";
}
}
}
```
4. 配置 mybatis 和数据库信息
在 application.properties 文件中添加以下配置:
```
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8&autoReconnect=true&failOverReadOnly=false&maxReconnects=10
spring.datasource.username=root
spring.datasource.password=root
mybatis.mapper-locations=classpath:mapper/*.xml
```
5. 创建 UserMapper 接口和 XML 映射文件
```java
@Mapper
public interface UserMapper {
User selectByUsernameAndPassword(@Param("username") String username, @Param("password") String password);
}
```
在 mapper 文件夹下创建 UserMapper.xml 文件,编写 SQL 语句:
```xml
<mapper namespace="com.example.demo.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.example.demo.entity.User">
<id column="username" property="username" />
<result column="password" property="password" />
</resultMap>
<select id="selectByUsernameAndPassword" resultMap="BaseResultMap">
select * from user where username=#{username} and password=#{password}
</select>
</mapper>
```
6. 编写测试代码
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserControllerTests {
@Autowired
private UserController userController;
@Test
public void testLogin() {
User user = new User();
user.setUsername("admin");
user.setPassword("123456");
String result = userController.login(user);
assertEquals("登录成功", result);
}
}
```
以上就是一个基于 Spring Boot 的用户登录案例的实现过程,希望对你有所帮助。
@Controller public class LoginIndexAction { @Autowired LoginIndexManager loginIndexManager; @Autowired IndexManager indexManager; public IndexManager getIndexManager() { return indexManager; } public void setIndexManager(IndexManager indexManager) { this.indexManager = indexManager; } public LoginIndexManager getLoginIndexManager() { return loginIndexManager; } public void setLoginIndexManager(LoginIndexManager loginIndexManager) { this.loginIndexManager = loginIndexManager; } /** * @Title: InSystem * @Description: 用户登录 * @return String */ @RequestMapping(value="LoginInSystem.action",method=RequestMethod.POST) @ResponseBody public JSONData InSystem(User params, ModelMap model,HttpServletRequest request,HttpServletResponse response,HttpSession httpSession){ JSONData jsonData = new JSONData(); try { //用户登录查询 params.setUser_type(1); User admin = loginIndexManager.getUser(params); if (admin!=null) { httpSession.setAttribute("userFront", admin); }else { jsonData.setErrorReason("用户名或密码错误"); return jsonData; } } catch (Exception e) { e.printStackTrace(); jsonData.setErrorReason("登录异常,请稍后重试"); return jsonData; } return jsonData; 解释一下这段代码 }
这段代码是一个Java类的控制器,用于处理用户登录请求。该类使用了Spring框架的注解来标识依赖注入和请求处理方法。
@Controller注解表示这是一个控制器类,@Autowired注解表示该类依赖于其他类的实例,这里依赖了LoginIndexManager和IndexManager类的实例。这些实例由Spring框架自动注入,无需手动创建。
@RequestMapping注解标识了处理的请求路径和请求方法,这里处理的是POST请求,请求路径为“LoginInSystem.action”。
@ResponseBody注解表示返回值将被视为响应内容,而不是视图名称。
InSystem方法处理用户登录请求,接收一个User对象作为参数,该对象包含了用户输入的用户名和密码。该方法首先设置用户类型为1,表示前台用户。然后调用LoginIndexManager的getUser方法查询用户是否存在,如果用户存在,则将用户信息存储到session中。如果用户不存在,则返回一个包含错误信息的JSONData对象。
如果出现异常,则返回一个包含错误信息的JSONData对象。
最后,该方法返回一个JSONData对象,它包含了用户登录的结果信息。
阅读全文
相关推荐
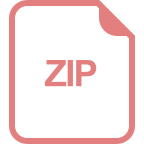
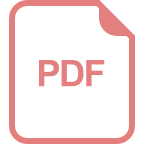
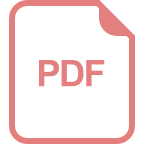
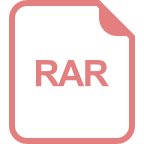
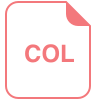
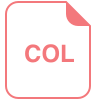
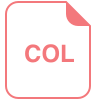
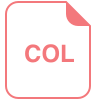
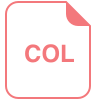
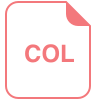
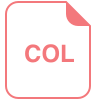
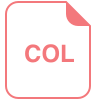
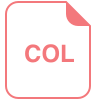



