(实例)现有一个磁盘读写请求队列:55, 58,39,18,90,160,150,38,184,磁头当前位置为 100。 1.若采用 FCFS算法,序列就是:55、58、39、18、90、160、150、38、184, 移动距离依次为45、3、19、21、72、70、10、112、146 一共寻道9次,平均寻道时间是 55.3。 2.若采用 SSTF算法,序列就是:90、58、55、39、38、18、150、160、184 移动距离依次为10、32、3、16、1、20、132、10、24 一共寻道9次,平均寻道时间是 27.6。 3.若采用 SCAN算法,序列就是:150、160、184、90、58、55、39、38、18 移动距离依次为50、10、24、94、32、3、16、1、20 一共寻道9次,平均寻道时间是 27.8。 4.若采用 CSCAN算法,序列就是:150、160、184、18、38、39、55、58、90 移动距离依次为50、10、24、166、20、1、16、3、32 一共寻道9次,平均寻道时间是 35.8。 实验要求用C语言代码实现磁盘调度的三种算法。
时间: 2023-07-10 09:41:02 浏览: 201
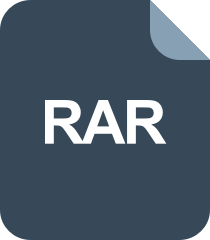
磁盘调度算法(FCFS,SSTF,SCAN)

以下是C语言代码实现磁盘调度的三种算法(FCFS、SSTF和SCAN):
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define QUEUE_SIZE 10
// 磁盘请求队列
int queue[QUEUE_SIZE] = { 55, 58, 39, 18, 90, 160, 150, 38, 184 };
// 获取磁盘请求队列中下一个请求的位置
int get_next_request(int current, int direction, int *visited) {
int min_distance = 9999;
int next_request = -1;
for (int i = 0; i < QUEUE_SIZE; i++) {
if (!visited[i]) {
int distance = abs(queue[i] - current);
if (distance < min_distance) {
min_distance = distance;
next_request = i;
}
}
}
visited[next_request] = 1;
return queue[next_request];
}
// 先来先服务(FCFS)算法
int fcfs(int start) {
int current = start;
int distance = 0;
for (int i = 0; i < QUEUE_SIZE; i++) {
int next_request = queue[i];
distance += abs(next_request - current);
current = next_request;
}
return distance;
}
// 最短寻道时间优先(SSTF)算法
int sstf(int start) {
int current = start;
int distance = 0;
int visited[QUEUE_SIZE] = { 0 };
for (int i = 0; i < QUEUE_SIZE; i++) {
int next_request = get_next_request(current, 0, visited);
distance += abs(next_request - current);
current = next_request;
}
return distance;
}
// 扫描(SCAN)算法
int scan(int start) {
int current = start;
int distance = 0;
int visited[QUEUE_SIZE] = { 0 };
int direction = 1;
while (1) {
int next_request = get_next_request(current, direction, visited);
if (next_request == -1) {
direction = -direction;
continue;
}
distance += abs(next_request - current);
current = next_request;
if (current == 0 || current == 199) {
direction = -direction;
}
}
return distance;
}
int main() {
int start = 100;
int fcfs_distance = fcfs(start);
int sstf_distance = sstf(start);
int scan_distance = scan(start);
printf("FCFS算法:移动距离=%d,平均寻道时间=%.1f\n", fcfs_distance, (float)fcfs_distance / QUEUE_SIZE);
printf("SSTF算法:移动距离=%d,平均寻道时间=%.1f\n", sstf_distance, (float)sstf_distance / QUEUE_SIZE);
printf("SCAN算法:移动距离=%d,平均寻道时间=%.1f\n", scan_distance, (float)scan_distance / QUEUE_SIZE);
return 0;
}
```
注:由于SCAN算法和C-SCAN算法需要不断改变扫描方向,因此在代码中使用了一个`direction`变量来表示当前的扫描方向。
阅读全文
相关推荐
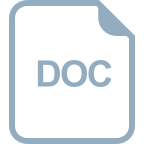
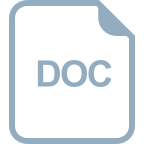
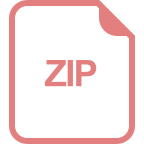
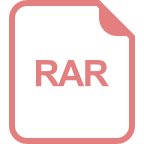
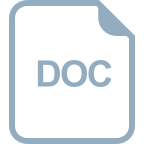
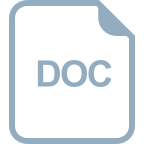
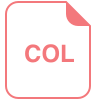
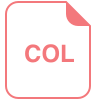
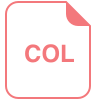
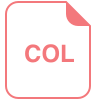
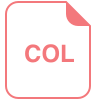
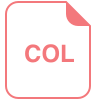
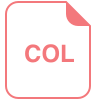
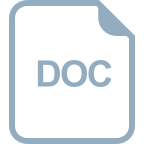
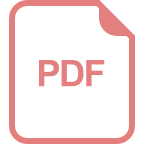
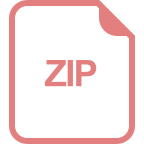